Image Geometry Transforms Functions
Routines manipulating an image geometry.
These functions can be found in the nppig library. Linking to only the sub-libraries that you use can significantly save link time, application load time, and CUDA runtime startup time when using dynamic libraries.
Geometric Transform API Specifics
This section covers some of the unique API features common to the geometric transform primitives.
Geometric Transforms and ROIs
Geometric transforms operate on source and destination ROIs. The way these ROIs affect the processing of pixels differs from other (non geometric) image-processing primitives: Only pixels in the intersection of the destination ROI and the transformed source ROI are being processed.
The typical processing proceedes as follows:
Transform the rectangular source ROI (given in source image coordinates) into the destination image space. This yields a quadrilateral.
Write only pixels in the intersection of the transformed source ROI and the destination ROI.
Pixel Interpolation
The majority of image geometry transform operation need to perform a resampling of the source image as source and destination pixels are not coincident.
NPP supports the following pixel inerpolation modes (in order from fastest to slowest and lowest to highest quality):
nearest neighbor
linear interpolation
cubic convolution
supersampling
interpolation using Lanczos window function
Resize Error Codes
The resize primitives return the following error codes:
- NPP_WRONG_INTERSECTION_ROI_ERROR indicates an error condition if
srcROIRect has no intersection with the source image.
- NPP_RESIZE_NO_OPERATION_ERROR if either destination ROI width or
height is less than 1 pixel.
- NPP_RESIZE_FACTOR_ERROR Indicates an error condition if either nXFactor or
nYFactor is less than or equal to zero or in the case of NPPI_INTER_SUPER are not both downscaling.
- NPP_INTERPOLATION_ERROR if eInterpolation has an illegal value.
- NPP_SIZE_ERROR if source size width or height is less than 2 pixels.
- NPP_RECTANGLE_ERROR if either destination ROI width or
height is less than 1 pixel.
ResizeSqrPixel
ResizeSqrPixel functions attempt to choose source pixels that would approximately represent the center of the destination pixels. It does so by using the following scaling formula to select source pixels for interpolation:
nAdjustedXFactor = 1.0 / nXFactor;
nAdjustedYFactor = 1.0 / nYFactor;
nAdjustedXShift = nXShift * nAdjustedXFactor + ((1.0 - nAdjustedXFactor) * 0.5);
nAdjustedYShift = nYShift * nAdjustedYFactor + ((1.0 - nAdjustedYFactor) * 0.5);
nSrcX = nAdjustedXFactor * nDstX - nAdjustedXShift;
nSrcY = nAdjustedYFactor * nDstY - nAdjustedYShift;
ResizeSqrPixel functions support the following interpolation modes:
NPPI_INTER_NN
NPPI_INTER_LINEAR
NPPI_INTER_CUBIC
NPPI_INTER_CUBIC2P_BSPLINE
NPPI_INTER_CUBIC2P_CATMULLROM
NPPI_INTER_CUBIC2P_B05C03
NPPI_INTER_SUPER
NPPI_INTER_LANCZOS
In the ResizeSqrPixel functions below source image clip checking is handled as follows:
If the source pixel fractional x and y coordinates are greater than or equal to oSizeROI.x and less than oSizeROI.x + oSizeROI.width and greater than or equal to oSizeROI.y and less than oSizeROI.y + oSizeROI.height then the source pixel is considered to be within the source image clip rectangle and the source image is sampled. Otherwise the source image is not sampled and a destination pixel is not written to the destination image.
GetResizeRect
Returns NppiRect which represents the offset and size of the destination rectangle that would be generated by resizing the source NppiRect by the requested scale factors and shifts.
-
NppStatus nppiGetResizeRect(NppiRect oSrcROI, NppiRect *pDstRect, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
- Parameters
oSrcROI – Region of interest in the source image.
pDstRect – User supplied host memory pointer to an NppiRect structure that will be filled in by this function with the region of interest in the destination image.
nXFactor – Factor by which x dimension is changed.
nYFactor – Factor by which y dimension is changed.
nXShift – Source pixel shift in x-direction.
nYShift – Source pixel shift in y-direction.
eInterpolation – The type of eInterpolation to perform resampling.
- Returns
Image Data Related Error Codes, ROI Related Error Codes, Resize Error Codes
ResizeSqrPixel
Resizes images.
Common parameters for nppiResizeSqrPackedPixel functions:
- param pSrc
- param nSrcStep
- param oSrcSize
Size in pixels of the source image.
- param oSrcROI
Region of interest in the source image.
- param pDst
- param nDstStep
- param oDstROI
Region of interest in the destination image.
- param nXFactor
Factor by which x dimension is changed.
- param nYFactor
Factor by which y dimension is changed.
- param nXShift
Source pixel shift in x-direction.
- param nYShift
Source pixel shift in y-direction.
- param eInterpolation
The type of eInterpolation to perform resampling.
- param nppStreamCtx
Common parameters for nppiResizeSqrPlanarPixel functions:
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nSrcStep
- param oSrcSize
Size in pixels of the source image.
- param oSrcROI
Region of interest in the source image.
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nDstStep
- param oDstROI
Region of interest in the destination image.
- param nXFactor
Factor by which x dimension is changed.
- param nYFactor
Factor by which y dimension is changed.
- param nXShift
Source pixel shift in x-direction.
- param nYShift
Source pixel shift in y-direction.
- param eInterpolation
The type of eInterpolation to perform resampling.
- param nppStreamCtx
-
NppStatus nppiResizeSqrPixel_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
1 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 8-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_P3R_Ctx(const Npp8u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_P3R(const Npp8u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_P4R_Ctx(const Npp8u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_8u_P4R(const Npp8u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
1 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 16-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_P3R_Ctx(const Npp16u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_P3R(const Npp16u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_P4R_Ctx(const Npp16u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16u_P4R(const Npp16u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_C1R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_C1R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
1 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_C3R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_C3R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_C4R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_C4R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_AC4R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_AC4R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 16-bit signed image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_P3R_Ctx(const Npp16s *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_P3R(const Npp16s *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_P4R_Ctx(const Npp16s *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_16s_P4R(const Npp16s *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16s *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
1 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 32-bit floating point image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_P3R_Ctx(const Npp32f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_P3R(const Npp32f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_P4R_Ctx(const Npp32f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_32f_P4R(const Npp32f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_C1R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 64-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_C1R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
1 channel 64-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_C3R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 64-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_C3R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 64-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_C4R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 64-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_C4R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 64-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_AC4R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 64-bit floating point image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_AC4R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 64-bit floating point image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeSqrPackedPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_P3R_Ctx(const Npp64f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 64-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_P3R(const Npp64f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst[3], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
3 channel 64-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_P4R_Ctx(const Npp64f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 64-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeSqrPixel_64f_P4R(const Npp64f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst[4], int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, double nXShift, double nYShift, int eInterpolation)
4 channel 64-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResizeSqrPlanarPixel functions:.
-
NppStatus nppiResizeAdvancedGetBufferHostSize_8u_C1R(NppiSize oSrcROI, NppiSize oDstROI, int *hpBufferSize, int eInterpolationMode)
Buffer size for nppiResizeSqrPixel_8u_C1R_Advanced.
- Parameters
oSrcROI – Region-Of-Interest (ROI).
oDstROI – Region-Of-Interest (ROI).
hpBufferSize – Required buffer size. Important: hpBufferSize is a host pointer. Scratch Buffer and Host Pointer.
eInterpolationMode – The type of eInterpolation to perform resampling. Currently only supports NPPI_INTER_LANCZOS3_Advanced.
- Returns
NPP_NULL_POINTER_ERROR if hpBufferSize is 0 (NULL), ROI Related Error Codes.
-
NppStatus nppiResizeSqrPixel_8u_C1R_Advanced_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, Npp8u *pBuffer, int eInterpolationMode, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned image resize.
This primitive matches the behavior of GraphicsMagick++.
- Parameters
pSrc – Source-Image Pointer.
nSrcStep – Source-Image Line Step.
oSrcSize – Size in pixels of the source image.
oSrcROI – Region of interest in the source image.
pDst – Destination-Image Pointer.
nDstStep – Destination-Image Line Step.
oDstROI – Region of interest in the destination image.
nXFactor – Factor by which x dimension is changed.
nYFactor – Factor by which y dimension is changed.
pBuffer – Device buffer that is used during calculations.
eInterpolationMode – The type of eInterpolation to perform resampling. Currently only supports NPPI_INTER_LANCZOS3_Advanced.
nppStreamCtx – Application Managed Stream Context.
- Returns
Image Data Related Error Codes, ROI Related Error Codes, Resize Error Codes
-
NppStatus nppiResizeSqrPixel_8u_C1R_Advanced(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, double nXFactor, double nYFactor, Npp8u *pBuffer, int eInterpolationMode)
1 channel 8-bit unsigned image resize.
This primitive matches the behavior of GraphicsMagick++.
- Parameters
pSrc – Source-Image Pointer.
nSrcStep – Source-Image Line Step.
oSrcSize – Size in pixels of the source image.
oSrcROI – Region of interest in the source image.
pDst – Destination-Image Pointer.
nDstStep – Destination-Image Line Step.
oDstROI – Region of interest in the destination image.
nXFactor – Factor by which x dimension is changed.
nYFactor – Factor by which y dimension is changed.
pBuffer – Device buffer that is used during calculations.
eInterpolationMode – The type of eInterpolation to perform resampling. Currently only supports NPPI_INTER_LANCZOS3_Advanced.
- Returns
Image Data Related Error Codes, ROI Related Error Codes, Resize Error Codes
Resize
Resize functions use scale factor automatically determined by the width and height ratios of input and output Region-Of-Interest (ROI).
This simplified function replaces the previous version which was deprecated in an earlier release. In this function the resize scale factor is automatically determined by the width and height ratios of oSrcRectROI and oDstRectROI. If either of those parameters intersect their respective image sizes then pixels outside the image size width and height will not be processed.
Resize supports the following interpolation modes:
NPPI_INTER_NN
NPPI_INTER_LINEAR
NPPI_INTER_CUBIC
NPPI_INTER_SUPER
NPPI_INTER_LANCZOS
The resize primitives return the following error codes: Resize Error Codes.
GetResizeTiledSourceOffset
Helper function that can be used when tiling a destination image with a source image using multiple Resize calls.
oSrcRectROI and oDstRectROI widths and heights should remain unmodified even if they will overlap source and destination image sizes. oDstRectROI offsets should be set to the destination offset of the new tile. Resize function processing will stop when source or destination image sizes are reached, any unavailable source image pixels beyond source image size will be border replicated. There is no particular association assumed between source and destination image locations. The values of oSrcRectROI.x and oSrcRectROI.y are ignored during this function call.
-
NppStatus nppiGetResizeTiledSourceOffset(NppiRect oSrcRectROI, NppiRect oDstRectROI, NppiPoint *pNewSrcRectOffset)
- Parameters
oSrcRectROI – Region of interest in the source image (may overlap source image size width and height).
oDstRectROI – Region of interest in the destination image (may overlap destination image size width and height).
pNewSrcRectOffset – Pointer to host memory NppiPoint object that will contain the new source image ROI offset to be used in the nppiResize call to generate that tile.
- Returns
Image Data Related Error Codes, ROI Related Error Codes, Resize Error Codes
Resize
Resizes images.
Common parameters for nppiResize packed pixel functions:
- param pSrc
Source-Image Pointer to origin of source image.
- param nSrcStep
- param oSrcSize
Size in pixels of the entire source image.
- param oSrcRectROI
Region of interest in the source image (may overlap source image size width and height).
- param pDst
Destination-Image Pointer to origin of destination image.
- param nDstStep
- param oDstSize
Size in pixels of the entire destination image.
- param oDstRectROI
Region of interest in the destination image (may overlap destination image size width and height).
- param eInterpolation
The type of eInterpolation to perform resampling (16f versions do not support Lanczos interpolation).
- param nppStreamCtx
Common parameters for nppiResize planar pixel functions:
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane origin pointers).
- param nSrcStep
- param oSrcSize
Size in pixels of the entire source image.
- param oSrcRectROI
Region of interest in the source image (may overlap source image size width and height).
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane origin pointers).
- param nDstStep
- param oDstSize
Size in pixels of the entire destination image.
- param oDstRectROI
Region of interest in the destination image (may overlap destination image size width and height).
- param eInterpolation
The type of eInterpolation to perform resampling.
- param nppStreamCtx
-
NppStatus nppiResize_8u_C1R_Ctx(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_C1R(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
1 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_C3R_Ctx(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_C3R(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_C4R_Ctx(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_C4R(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 8-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_AC4R_Ctx(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_AC4R(const Npp8u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 8-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_8u_P3R_Ctx(const Npp8u *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_8u_P3R(const Npp8u *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_8u_P4R_Ctx(const Npp8u *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_8u_P4R(const Npp8u *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp8u *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 8-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16u_C1R_Ctx(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_C1R(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
1 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_C3R_Ctx(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_C3R(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_C4R_Ctx(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_C4R(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit unsigned image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_AC4R_Ctx(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_AC4R(const Npp16u *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit unsigned image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16u_P3R_Ctx(const Npp16u *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16u_P3R(const Npp16u *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16u_P4R_Ctx(const Npp16u *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16u_P4R(const Npp16u *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16u *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit unsigned planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16s_C1R_Ctx(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_C1R(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
1 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_C3R_Ctx(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_C3R(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_C4R_Ctx(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_C4R(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit signed image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_AC4R_Ctx(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_AC4R(const Npp16s *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit signed image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16s_P3R_Ctx(const Npp16s *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16s_P3R(const Npp16s *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16s_P4R_Ctx(const Npp16s *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16s_P4R(const Npp16s *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16s *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit signed planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_16f_C1R_Ctx(const Npp16f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16f_C1R(const Npp16f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
1 channel 16-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16f_C3R_Ctx(const Npp16f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16f_C3R(const Npp16f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 16-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16f_C4R_Ctx(const Npp16f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_16f_C4R(const Npp16f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp16f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 16-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_C1R_Ctx(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_C1R(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
1 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_C3R_Ctx(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_C3R(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_C4R_Ctx(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_C4R(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 32-bit floating point image resize.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_AC4R_Ctx(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_AC4R(const Npp32f *pSrc, int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst, int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 32-bit floating point image resize not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResize packed pixel functions:.
-
NppStatus nppiResize_32f_P3R_Ctx(const Npp32f *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_32f_P3R(const Npp32f *pSrc[3], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst[3], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
3 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_32f_P4R_Ctx(const Npp32f *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
-
NppStatus nppiResize_32f_P4R(const Npp32f *pSrc[4], int nSrcStep, NppiSize oSrcSize, NppiRect oSrcRectROI, Npp32f *pDst[4], int nDstStep, NppiSize oDstSize, NppiRect oDstRectROI, int eInterpolation)
4 channel 32-bit floating point planar image resize.
For common parameter descriptions, see Common parameters for nppiResize planar pixel functions:.
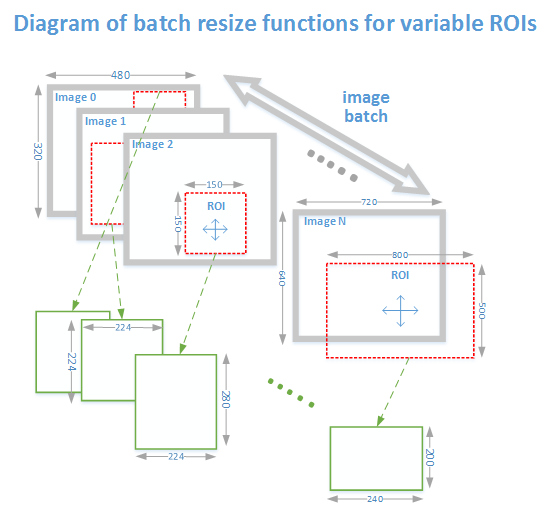
ResizeBatch
Above is the diagram of batch resize functions for variable ROIs. Figure shows the flexibility that the API can handle. The ROIs for source and destination images can be any rectangular width and height that reflects the needed resize factors, inside or beyond the image boundary.
ResizeBatch functions use scale factor automatically determined by the width and height ratios for each pair of input / output images in provided batches.
In this function as in nppiResize the resize scale factor is automatically determined by the width and height ratios of oSrcRectROI and oDstRectROI. If either of those parameters intersect their respective image sizes then pixels outside the image size width and height will not be processed. Details of the resize operation are described above in the Resize section. ResizeBatch generally takes the same parameter list as Resize except that there is a list of N instances of those parameters (N > 1) and that list is passed in device memory. A convenient data structure is provided that allows for easy initialization of the parameter lists. The only restriction on these functions is that there is one single source ROI rectangle and one single destination ROI rectangle which are applied respectively to each image in the batch. The primary purpose of this function is to provide improved performance for batches of smaller images as long as GPU resources are available. Therefore it is recommended that the function not be used for very large images as there may not be resources available for processing several large images simultaneously.
A single set of oSrcRectROI and oDstRectROI values are applied to each source image and destination image in the batch in the nppiResizeBatch version of the function while per image specific oSrcRectROI and oDstRectROI values can be used in the nppiResizeBatch_Advanced version of the function. Source and destination image sizes may vary but oSmallestSrcSize and oSmallestDstSize must be set to the smallest source and destination image sizes in the batch. The parameters in the
NppiResizeBatchCXR structure represent the corresponding per-image nppiResize parameters for each image in the nppiResizeBatch functions and the NppiImageDescriptor and NppiResizeBatchROI_Advanced structures represent the corresponding per-image nppiResize parameters for the nppiResizeBatch_Advanced functions. The NppiResizeBatchCXR or NppiImageDescriptor and NppiResizeBatchROI_Advanced arrays must be in device memory.ResizeBatch supports the following interpolation modes:
NPPI_INTER_NN
NPPI_INTER_LINEAR
NPPI_INTER_CUBIC
NPPI_INTER_SUPER
The resize primitives return the following error codes: Resize Error Codes.
Common parameters for nppiResizeBatch functions:
Common parameters for nppiResizeBatchAdvanced functions:
- param oSmallestSrcSize
Size in pixels of the entire smallest source image width and height, may be from different images.
- param oSrcRectROI
Region of interest in the source images (may overlap source image size width and height).
- param oSmallestDstSize
Size in pixels of the entire smallest destination image width and height, may be from different images.
- param oDstRectROI
Region of interest in the destination images (may overlap destination image size width and height).
- param eInterpolation
The type of eInterpolation to perform resampling. Currently limited to NPPI_INTER_NN, NPPI_INTER_LINEAR, NPPI_INTER_CUBIC, or NPPI_INTER_SUPER.
- param pBatchList
Device memory pointer to nBatchSize list of NppiResizeBatchCXR structures.
- param pBatchSrc
Device pointer to NppiImageDescriptor list of source image descriptors. User needs to intialize this structure and copy it to device.
- param pBatchDst
Device pointer to NppiImageDescriptor list of destination image descriptors. User needs to intialize this structure and copy it to device.
- param pBatchROI
Device pointer to NppiResizeBatchROI_Advanced list of per-image variable ROIs. User needs to initialize this structure and copy it to device.
- param nBatchSize
Number of NppiResizeBatchCXR structures in this call (must be > 1).
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Resize Error Codes
- param nMaxWidth
The maximum width of all destination ROIs
- param nMaxHeight
The maximum height of all destination ROIs
- param pBatchSrc
Device pointer to NppiImageDescriptor list of source image descriptors. User needs to intialize this structure and copy it to device.
- param pBatchDst
Device pointer to NppiImageDescriptor list of destination image descriptors. User needs to intialize this structure and copy it to device.
- param pBatchROI
Device pointer to NppiResizeBatchROI_Advanced list of per-image variable ROIs. User needs to initialize this structure and copy it to device.
- param nBatchSize
Number of images in a batch.
- param eInterpolation
The type of eInterpolation to perform resampling.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Resize Error Codes
Functions
-
NppStatus nppiResizeBatch_8u_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 8-bit image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 8-bit image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 8-bit image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 8-bit image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 8-bit image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 8-bit image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_AC4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 8-bit image resize batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_AC4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 8-bit image resize batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 32-bit floating point image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 32-bit floating point image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 32-bit floating point image resize batch.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_AC4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_32f_AC4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiSize oSmallestDstSize, NppiRect oDstRectROI, int eInterpolation, NppiResizeBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 32-bit floating point image resize batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatch functions:.
-
NppStatus nppiResizeBatch_8u_C1R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 8-bit image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_C1R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
1 channel 8-bit image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_C3R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_C3R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
3 channel 8-bit image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_C4R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_C4R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
3 channel 8-bit image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_AC4R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit image resize batch for variable ROI not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_8u_AC4R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
4 channel 8-bit image resize batch for variable ROI not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_16f_C1R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_16f_C1R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
1 channel 16-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_16f_C3R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_16f_C3R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
3 channel 16-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_16f_C4R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_16f_C4R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
4 channel 16-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_C1R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_C1R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
1 channel 32-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_C3R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_C3R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
3 channel 32-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_C4R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_C4R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
4 channel 32-bit floating point image resize batch for variable ROI.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_AC4R_Advanced_Ctx(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image resize batch for variable ROI not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
-
NppStatus nppiResizeBatch_32f_AC4R_Advanced(int nMaxWidth, int nMaxHeight, NppiImageDescriptor *pBatchSrc, NppiImageDescriptor *pBatchDst, NppiResizeBatchROI_Advanced *pBatchROI, unsigned int nBatchSize, int eInterpolation)
4 channel 32-bit floating point image resize batch for variable ROI not affecting alpha.
For common parameter descriptions, see Common parameters for nppiResizeBatchAdvanced functions:.
Remap
Routines providing remap functionality.
Remap supports the following interpolation modes:
NPPI_INTER_NN NPPI_INTER_LINEAR NPPI_INTER_CUBIC NPPI_INTER_CUBIC2P_BSPLINE NPPI_INTER_CUBIC2P_CATMULLROM NPPI_INTER_CUBIC2P_B05C03 NPPI_INTER_LANCZOS
Remap chooses source pixels using pixel coordinates explicitely supplied in two 2D device memory image arrays pointed to by the pXMap and pYMap pointers. The pXMap array contains the X coordinated and the pYMap array contains the Y coordinate of the corresponding source image pixel to use as input. These coordinates are in floating point format so fraction pixel positions can be used. The coordinates of the source pixel to sample are determined as follows:
nSrcX = pxMap[nDstX, nDstY] nSrcY = pyMap[nDstX, nDstY]
In the Remap functions below source image clip checking is handled as follows:
If the source pixel fractional x and y coordinates are greater than or equal to oSizeROI.x and less than oSizeROI.x + oSizeROI.width and greater than or equal to oSizeROI.y and less than oSizeROI.y + oSizeROI.height then the source pixel is considered to be within the source image clip rectangle and the source image is sampled. Otherwise the source image is not sampled and a destination pixel is not written to the destination image.
Error Codes
The remap primitives return the following error codes:
- NPP_WRONG_INTERSECTION_ROI_ERROR indicates an error condition if
srcROIRect has no intersection with the source image.
- NPP_INTERPOLATION_ERROR if eInterpolation has an illegal value.
Common parameters for nppiRemap packed pixel functions:
Common parameters for nppiRemap planar pixel functions:
- param pSrc
- param nSrcStep
- param oSrcSize
Size in pixels of the source image.
- param oSrcROI
Region of interest in the source image.
- param pXMap
Device memory pointer to 2D image array of X coordinate values to be used when sampling source image.
- param nXMapStep
pXMap image array line step in bytes.
- param pYMap
Device memory pointer to 2D image array of Y coordinate values to be used when sampling source image.
- param nYMapStep
pYMap image array line step in bytes.
- param pDst
- param nDstStep
- param oDstSizeROI
Region of interest size in the destination image.
- param eInterpolation
The type of eInterpolation to perform resampling.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Error Codes
- param pSrc
- param nSrcStep
- param oSrcSize
Size in pixels of the source image.
- param oSrcROI
Region of interest in the source image.
- param pXMap
Device memory pointer to 2D image array of X coordinate values to be used when sampling source image.
- param nXMapStep
pXMap image array line step in bytes.
- param pYMap
Device memory pointer to 2D image array of Y coordinate values to be used when sampling source image.
- param nYMapStep
pYMap image array line step in bytes.
- param pDst
- param nDstStep
- param oDstSizeROI
Region of interest size in the destination image.
- param eInterpolation
The type of eInterpolation to perform resampling.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Error Codes
Remap
Remaps images.
-
NppStatus nppiRemap_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
1 channel 8-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 8-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 8-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 8-bit unsigned image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_8u_P3R_Ctx(const Npp8u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_8u_P3R(const Npp8u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 8-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_8u_P4R_Ctx(const Npp8u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_8u_P4R(const Npp8u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp8u *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 8-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
1 channel 16-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 16-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 16-bit unsigned image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 16-bit unsigned image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16u_P3R_Ctx(const Npp16u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16u_P3R(const Npp16u *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 16-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16u_P4R_Ctx(const Npp16u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16u_P4R(const Npp16u *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16u *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 16-bit unsigned planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16s_C1R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 16-bit signed image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_C1R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
1 channel 16-bit signed image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_C3R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit signed image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_C3R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 16-bit signed image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_C4R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_C4R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 16-bit signed image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_AC4R_Ctx(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_AC4R(const Npp16s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 16-bit signed image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_16s_P3R_Ctx(const Npp16s *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 16-bit signed planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16s_P3R(const Npp16s *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 16-bit signed planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16s_P4R_Ctx(const Npp16s *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 16-bit signed planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_16s_P4R(const Npp16s *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp16s *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 16-bit signed planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
1 channel 32-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 32-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 32-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 32-bit floating point image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_32f_P3R_Ctx(const Npp32f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_32f_P3R(const Npp32f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 32-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_32f_P4R_Ctx(const Npp32f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_32f_P4R(const Npp32f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp32f *pXMap, int nXMapStep, const Npp32f *pYMap, int nYMapStep, Npp32f *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 32-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_64f_C1R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
1 channel 64-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_C1R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
1 channel 64-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_C3R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 64-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_C3R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 64-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_C4R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 64-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_C4R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 64-bit floating point image remap.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_AC4R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 64-bit floating point image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_AC4R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst, int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 64-bit floating point image remap not affecting alpha.
For common parameter descriptions, see Common parameters for nppiRemap packed pixel functions:.
-
NppStatus nppiRemap_64f_P3R_Ctx(const Npp64f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
3 channel 64-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_64f_P3R(const Npp64f *const pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst[3], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
3 channel 64-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_64f_P4R_Ctx(const Npp64f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation, NppStreamContext nppStreamCtx)
4 channel 64-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
-
NppStatus nppiRemap_64f_P4R(const Npp64f *const pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const Npp64f *pXMap, int nXMapStep, const Npp64f *pYMap, int nYMapStep, Npp64f *pDst[4], int nDstStep, NppiSize oDstSizeROI, int eInterpolation)
4 channel 64-bit floating point planar image remap.
For common parameter descriptions, see Common parameters for nppiRemap planar pixel functions:.
Rotate
Rotates an image around the origin (0,0) and then shifts it.
Rotate Error Codes
NPP_INTERPOLATION_ERROR if eInterpolation has an illegal value.
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1.
NPP_WRONG_INTERSECTION_ROI_ERROR indicates an error condition if srcROIRect has no intersection with the source image.
NPP_WRONG_INTERSECTION_QUAD_WARNING indicates a warning that no operation is performed if the transformed source ROI does not intersect the destination ROI.
Rotate Utility Functions
The set of rotate utility functions.
Functions
-
NppStatus nppiGetRotateQuad(NppiRect oSrcROI, double aQuad[4][2], double nAngle, double nShiftX, double nShiftY)
Compute shape of rotated image.
- Parameters
oSrcROI – Region-of-interest of the source image.
aQuad – Array of 2D points. These points are the locations of the corners of the rotated ROI.
nAngle – The rotation nAngle.
nShiftX – Post-rotation shift in x-direction
nShiftY – Post-rotation shift in y-direction
- Returns
-
NppStatus nppiGetRotateBound(NppiRect oSrcROI, double aBoundingBox[2][2], double nAngle, double nShiftX, double nShiftY)
Compute bounding-box of rotated image.
- Parameters
oSrcROI – Region-of-interest of the source image.
aBoundingBox – Two 2D points representing the bounding-box of the rotated image. All four points from nppiGetRotateQuad are contained inside the axis-aligned rectangle spanned by the the two points of this bounding box.
nAngle – The rotation angle.
nShiftX – Post-rotation shift in x-direction.
nShiftY – Post-rotation shift in y-direction.
- Returns
Mirror
Mirrors images horizontally, vertically or diagonally.
Mirror Error Codes
NPP_MIRROR_FLIP_ERROR if flip has an illegal value.
NPP_SIZE_ERROR if in_place ROI width or height are not even numbers.
Common parameters for nppiMirror functions:
- param pSrcDst
In-Place Image Pointer for inplace functions.
- param nSrcDstStep
In-Place-Image Line Step for inplace functions.
- param pSrc
Source-Image Pointer for non-inplace functions.
- param nSrcStep
Source-Image Line Step for non-inplace functions.
- param pDst
Destination-Image Pointer for non-inplace functions.
- param nDstStep
Destination-Image Line Step for non-inplace functions.
- param oROI
Region-Of-Interest (ROI) (in_place ROI widths and heights must be even numbers).
- param flip
Specifies the axis about which the image is to be mirrored.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Mirror Error Codes
Functions
-
NppStatus nppiMirror_8u_C1R_Ctx(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C1R(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
1 channel 8-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C1IR_Ctx(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C1IR(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
1 channel 8-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C3R_Ctx(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C3R(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
3 channel 8-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C3IR_Ctx(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C3IR(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
3 channel 8-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C4R_Ctx(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C4R(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 8-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C4IR_Ctx(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_C4IR(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 8-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_AC4R_Ctx(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_AC4R(const Npp8u *pSrc, int nSrcStep, Npp8u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 8-bit unsigned image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_AC4IR_Ctx(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_8u_AC4IR(Npp8u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 8-bit unsigned in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C1R_Ctx(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 16-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C1R(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
1 channel 16-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C1IR_Ctx(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 16-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C1IR(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
1 channel 16-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C3R_Ctx(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C3R(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
3 channel 16-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C3IR_Ctx(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 16-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C3IR(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
3 channel 16-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C4R_Ctx(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C4R(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit unsigned image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C4IR_Ctx(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_C4IR(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit unsigned in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_AC4R_Ctx(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_AC4R(const Npp16u *pSrc, int nSrcStep, Npp16u *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit unsigned image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_AC4IR_Ctx(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit unsigned in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16u_AC4IR(Npp16u *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit unsigned in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C1R_Ctx(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 16-bit signed image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C1R(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
1 channel 16-bit signed image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C1IR_Ctx(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 16-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C1IR(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
1 channel 16-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C3R_Ctx(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 16-bit signed image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C3R(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
3 channel 16-bit signed image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C3IR_Ctx(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 16-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C3IR(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
3 channel 16-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C4R_Ctx(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C4R(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit signed image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C4IR_Ctx(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_C4IR(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_AC4R_Ctx(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit signed image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_AC4R(const Npp16s *pSrc, int nSrcStep, Npp16s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit signed image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_AC4IR_Ctx(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 16-bit signed in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_16s_AC4IR(Npp16s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 16-bit signed in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C1R_Ctx(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 32-bit image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C1R(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
1 channel 32-bit image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C1IR_Ctx(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 32-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C1IR(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
1 channel 32-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C3R_Ctx(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 32-bit image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C3R(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
3 channel 32-bit image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C3IR_Ctx(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 32-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C3IR(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
3 channel 32-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C4R_Ctx(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C4R(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C4IR_Ctx(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_C4IR(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit signed in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_AC4R_Ctx(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_AC4R(const Npp32s *pSrc, int nSrcStep, Npp32s *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_AC4IR_Ctx(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit signed in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32s_AC4IR(Npp32s *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit signed in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C1R_Ctx(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 32-bit float image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C1R(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
1 channel 32-bit float image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C1IR_Ctx(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
1 channel 32-bit float in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C1IR(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
1 channel 32-bit float in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C3R_Ctx(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 32-bit float image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C3R(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
3 channel 32-bit float image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C3IR_Ctx(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
3 channel 32-bit float in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C3IR(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
3 channel 32-bit float in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C4R_Ctx(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit float image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C4R(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit float image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C4IR_Ctx(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit float in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_C4IR(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit float in place image mirror.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_AC4R_Ctx(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit float image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_AC4R(const Npp32f *pSrc, int nSrcStep, Npp32f *pDst, int nDstStep, NppiSize oROI, NppiAxis flip)
4 channel 32-bit float image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
-
NppStatus nppiMirror_32f_AC4IR_Ctx(Npp32f *pSrcDst, int nSrcDstStep, NppiSize oROI, NppiAxis flip, NppStreamContext nppStreamCtx)
4 channel 32-bit float in place image mirror not affecting alpha.
For common parameter descriptions, see Common parameters for nppiMirror functions:.
Affine Transforms
Affine Transforms
The set of affine transform functions available in the library.
Affine Transform Error Codes
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_WRONG_INTERSECTION_ROI_ERROR Indicates an error condition if oSrcROI has no intersection with the source image
NPP_INTERPOLATION_ERROR Indicates an error condition if interpolation has an illegal value
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
NPP_WRONG_INTERSECTION_QUAD_WARNING Indicates a warning that no operation is performed if the transformed source ROI has no intersection with the destination ROI
Affine Transform Utility Functions
The set of affine transform utility functions.
Functions
-
NppStatus nppiGetAffineTransform(NppiRect oSrcROI, const double aQuad[4][2], double aCoeffs[2][3])
Computes affine transform coefficients based on source ROI and destination quadrilateral.
The function computes the coefficients of an affine transformation that maps the given source ROI (axis aligned rectangle with integer coordinates) to a quadrilateral in the destination image.
An affine transform in 2D is fully determined by the mapping of just three vertices. This function’s API allows for passing a complete quadrilateral effectively making the prolem overdetermined. What this means in practice is, that for certain quadrilaterals it is not possible to find an affine transform that would map all four corners of the source ROI to the four vertices of that quadrilateral.
The function circumvents this problem by only looking at the first three vertices of the destination image quadrilateral to determine the affine transformation’s coefficients. If the destination quadrilateral is indeed one that cannot be mapped using an affine transformation the functions informs the user of this situation by returning a NPP_AFFINE_QUAD_INCORRECT_WARNING.
- Parameters
oSrcROI – The source ROI. This rectangle needs to be at least one pixel wide and high. If either width or hight are less than one an NPP_RECTANGLE_ERROR is returned.
aQuad – The destination quadrilateral.
aCoeffs – The resulting affine transform coefficients.
- Returns
Error codes:
NPP_SIZE_ERROR Indicates an error condition if any image dimension has zero or negative value
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
NPP_AFFINE_QUAD_INCORRECT_WARNING Indicates a warning when quad does not conform to the transform properties. Fourth vertex is ignored, internally computed coordinates are used instead
-
NppStatus nppiGetAffineQuad(NppiRect oSrcROI, double aQuad[4][2], const double aCoeffs[2][3])
Compute shape of transformed image.
This method computes the quadrilateral in the destination image that the source ROI is transformed into by the affine transformation expressed by the coefficients array (aCoeffs).
- Parameters
oSrcROI – The source ROI.
aQuad – The resulting destination quadrangle.
aCoeffs – The afine transform coefficients.
- Returns
Error codes:
NPP_SIZE_ERROR Indicates an error condition if any image dimension has zero or negative value
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
-
NppStatus nppiGetAffineBound(NppiRect oSrcROI, double aBound[2][2], const double aCoeffs[2][3])
Compute bounding-box of transformed image.
The method effectively computes the bounding box (axis aligned rectangle) of the transformed source ROI (see nppiGetAffineQuad()).
- Parameters
oSrcROI – The source ROI.
aBound – The resulting bounding box.
aCoeffs – The afine transform coefficients.
- Returns
Error codes:
NPP_SIZE_ERROR Indicates an error condition if any image dimension has zero or negative value
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
Affine Transform
Transforms (warps) an image based on an affine transform.
The affine transform is given as a \(2\times 3\) matrix C. A pixel location \((x, y)\) in the source image is mapped to the location \((x', y')\) in the destination image. The destination image coorodinates are computed as follows:
Common parameters for nppiWarpAffine packed pixel functions:
Common parameters for nppiWarpAffine planar pixel functions:
- param pSrc
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Affine transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Affine Transform Error Codes
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
Destination-Planar-Image Pointer Array. (host memory array containing device memory image plane pointers)
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Affine transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Affine Transform Error Codes
Functions
-
NppStatus nppiWarpAffine_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 8-bit unsigned affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_8u_P3R_Ctx(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_8u_P3R(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_8u_P4R_Ctx(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_8u_P4R(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 8-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 16-bit unsigned affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16u_P3R_Ctx(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_16u_P3R(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_16u_P4R_Ctx(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_16u_P4R(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 16-bit unsigned affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32s_C1R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_C1R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_C3R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_C3R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_C4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_C4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_AC4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_AC4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit signed affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32s_P3R_Ctx(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32s_P3R(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32s_P4R_Ctx(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32s_P4R(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 32-bit signed affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_16f_C1R_Ctx(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16f_C1R(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 16-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16f_C3R_Ctx(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16f_C3R(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 16-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16f_C4R_Ctx(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_16f_C4R(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 16-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit floating-point affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_32f_P3R_Ctx(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32f_P3R(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32f_P4R_Ctx(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_32f_P4R(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 32-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_64f_C1R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_C1R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_C3R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_C3R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_C4R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_C4R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_AC4R_Ctx(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 64-bit floating-point affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_AC4R(const Npp64f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 64-bit floating-point affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffine packed pixel functions:.
-
NppStatus nppiWarpAffine_64f_P3R_Ctx(const Npp64f *aSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *aDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_64f_P3R(const Npp64f *aSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *aDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_64f_P4R_Ctx(const Npp64f *aSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *aDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
-
NppStatus nppiWarpAffine_64f_P4R(const Npp64f *aSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp64f *aDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 64-bit floating-point affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffine planar pixel functions:.
Affine Transform Batch
Details of the warp affine operation are described above in the WarpAffine section. WarpAffineBatch generally takes the same parameter list as WarpAffine except that there is a list of N instances of those parameters (N > 1) and that list is passed in device memory. A convenient data structure is provided that allows for easy initialization of the parameter lists. The aTransformedCoeffs array is for internal use only and should not be directly initialized by the application. The only restriction on these functions is that there is one single source ROI rectangle and one single destination ROI rectangle which are applied respectively to each image in the batch. The primary purpose of this function is to provide improved performance for batches of smaller images as long as GPU resources are available. Therefore it is recommended that the function not be used for very large images as there may not be resources available for processing several large images simultaneously.
A single set of oSrcRectROI and oDstRectROI values are applied to each source image and destination image in the batch. Source and destination image sizes may vary but oSmallestSrcSize must be set to the smallest source and image size in the batch. The parameters in the
NppiWarpAffineBatchCXR structure represent the corresponding per-image nppiWarpAffine parameters for each image in the batch. The NppiWarpAffineBatchCXR array must be in device memory. The nppiWarpAffineBatchInit function MUST be called AFTER the application has initialized the array of NppiWarpAffineBatchCXR structures and BEFORE calling any of the nppiWarpAffineBatch functions to so that the aTransformedCoeffs array can be internally pre-initialized for each image in the batch. The batch size passed to nppiWarpAffineBatchInit must match the batch size passed to the corresponding warp affine batch function.WarpAffineBatch supports the following interpolation modes:
NPPI_INTER_NN
NPPI_INTER_LINEAR
NPPI_INTER_CUBIC
Codes
The warp affine primitives return the following error codes:
- NPP_RECTANGLE_ERROR if either destination ROI width or
height is less than 1 pixel.
- NPP_INTERPOLATION_ERROR if eInterpolation has an illegal value.
- NPP_SIZE_ERROR if source size width or height is less than 2 pixels.
Common parameters for nppiWarpAffineBatch functions:
- param oSmallestSrcSize
Size in pixels of the entire smallest source image width and height, may be from different images.
- param oSrcRectROI
Region of interest in the source images.
- param oDstRectROI
Region of interest in the destination images.
- param eInterpolation
The type of eInterpolation to perform resampling. Currently limited to NPPI_INTER_NN, NPPI_INTER_LINEAR, or NPPI_INTER_CUBIC.
- param pBatchList
Device memory pointer to nBatchSize list of NppiWarpAffineBatchCXR structures.
- param nBatchSize
Number of NppiWarpAffineBatchCXR structures in this call (must be > 1).
- param nppStreamCtx
- return
Functions
-
NppStatus nppiWarpAffineBatchInit_Ctx(NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
Initializes the aTransformdedCoeffs array in pBatchList for each image in the list.
MUST be called before calling the corresponding warp affine batch function whenever any of the transformation matrices in the list have changed.
- Parameters
pBatchList – Device memory pointer to nBatchSize list of NppiWarpAffineBatchCXR structures.
nBatchSize – Number of NppiWarpAffineBatchCXR structures in this call (must be > 1).
nppStreamCtx – Application Managed Stream Context.
-
NppStatus nppiWarpAffineBatchInit(NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
Initializes the aTransformdedCoeffs array in pBatchList for each image in the list.
MUST be called before calling the corresponding warp affine batch function whenever any of the transformation matrices in the list have changed.
- Parameters
pBatchList – Device memory pointer to nBatchSize list of NppiWarpAffineBatchCXR structures.
nBatchSize – Number of NppiWarpAffineBatchCXR structures in this call (must be > 1).
-
NppStatus nppiWarpAffineBatch_8u_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned integer image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 8-bit unsigned integer image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned integer image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 8-bit unsigned integer image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned integer image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 8-bit unsigned integer image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_AC4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned integer image warp affine batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_8u_AC4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 8-bit unsigned integer image warp affine batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_16f_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 16-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_16f_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 16-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_16f_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 16-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_16f_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 16-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_16f_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 16-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_16f_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 16-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 32-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 32-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 32-bit floating point image warp affine batch.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_AC4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image warp affine batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
-
NppStatus nppiWarpAffineBatch_32f_AC4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpAffineBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 32-bit floating point image warp affine batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpAffineBatch functions:.
Backwards Affine Transform
Transforms (warps) an image based on an affine transform.
The affine transform is given as a \(2\times 3\) matrix C. A pixel location \((x, y)\) in the source image is mapped to the location \((x', y')\) in the destination image. The destination image coorodinates fullfil the following properties:
Common parameters for nppiWarpAffineBack packed pixel functions:
Common parameters for nppiWarpAffineBack planar pixel functions:
- param pSrc
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Affine transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Affine Transform Error Codes
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Affine transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Affine Transform Error Codes
Functions
-
NppStatus nppiWarpAffineBack_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 8-bit unsigned integer backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_P3R_Ctx(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_P3R(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_P4R_Ctx(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_8u_P4R(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 8-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 16-bit unsigned integer backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_P3R_Ctx(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_P3R(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_P4R_Ctx(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_16u_P4R(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 16-bit unsigned integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_C1R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_C1R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_C3R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_C3R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_C4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_C4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_AC4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_AC4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit signed integer backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_P3R_Ctx(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_P3R(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_P4R_Ctx(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32s_P4R(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 32-bit signed integer backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Single-channel 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel 32-bit floating-point backwards affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack packed pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_P3R_Ctx(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_P3R(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Three-channel planar 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_P4R_Ctx(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
-
NppStatus nppiWarpAffineBack_32f_P4R(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[2][3], int eInterpolation)
Four-channel planar 32-bit floating-point backwards affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineBack planar pixel functions:.
Quad-Based Affine Transform
Transforms (warps) an image based on an affine transform.
The affine transform is computed such that it maps a quadrilateral in source image space to a quadrilateral in destination image space.
An affine transform is fully determined by the mapping of 3 discrete points. The following primitives compute an affine transformation matrix that maps the first three corners of the source quad are mapped to the first three vertices of the destination image quad. If the fourth vertices do not match the transform, an NPP_AFFINE_QUAD_INCORRECT_WARNING is returned by the primitive.
Common parameters for nppiWarpAffineQuad packed pixel functions:
Common parameters for nppiWarpAffineQuad planar pixel functions:
- param pSrc
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param aSrcQuad
Source quad.
- param pDst
- param nDstStep
- param oDstROI
Destination ROI.
- param aDstQuad
Destination quad.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Affine Transform Error Codes
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI
- param aSrcQuad
Source quad.
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nDstStep
- param oDstROI
Destination ROI
- param aDstQuad
Destination quad.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Affine Transform Error Codes
Functions
-
NppStatus nppiWarpAffineQuad_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 8-bit unsigned integer quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_P3R_Ctx(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_P3R(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_P4R_Ctx(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_8u_P4R(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 8-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 16-bit unsigned integer quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_P3R_Ctx(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_P3R(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_P4R_Ctx(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_16u_P4R(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 16-bit unsigned integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_C1R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_C1R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_C3R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_C3R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_C4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_C4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_AC4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_AC4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit signed integer quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_P3R_Ctx(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_P3R(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_P4R_Ctx(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32s_P4R(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 32-bit signed integer quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit floating-point quad-based affine warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad packed pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_P3R_Ctx(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_P3R(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_P4R_Ctx(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
-
NppStatus nppiWarpAffineQuad_32f_P4R(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 32-bit floating-point quad-based affine warp.
For common parameter descriptions, see Common parameters for nppiWarpAffineQuad planar pixel functions:.
Perspective Transforms
Perspective Transform
The set of perspective transform functions available in the library.
Perspective Transform Error Codes
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_WRONG_INTERSECTION_ROI_ERROR Indicates an error condition if oSrcROI has no intersection with the source image
NPP_INTERPOLATION_ERROR Indicates an error condition if interpolation has an illegal value
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
NPP_WRONG_INTERSECTION_QUAD_WARNING Indicates a warning that no operation is performed if the transformed source ROI has no intersection with the destination ROI
The set of perspective transform utility functions.
Functions
-
NppStatus nppiGetPerspectiveTransform(NppiRect oSrcROI, const double quad[4][2], double aCoeffs[3][3])
Calculates perspective transform coefficients given source rectangular ROI and its destination quadrangle projection.
- Parameters
oSrcROI – Source ROI
quad – Destination quadrangle
aCoeffs – Perspective transform coefficients
- Returns
Error codes:
NPP_SIZE_ERROR Indicates an error condition if any image dimension has zero or negative value
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
-
NppStatus nppiGetPerspectiveQuad(NppiRect oSrcROI, double quad[4][2], const double aCoeffs[3][3])
Calculates perspective transform projection of given source rectangular ROI.
- Parameters
oSrcROI – Source ROI
quad – Destination quadrangle
aCoeffs – Perspective transform coefficients
- Returns
Error codes:
NPP_SIZE_ERROR Indicates an error condition if any image dimension has zero or negative value
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
-
NppStatus nppiGetPerspectiveBound(NppiRect oSrcROI, double bound[2][2], const double aCoeffs[3][3])
Calculates bounding box of the perspective transform projection of the given source rectangular ROI.
- Parameters
oSrcROI – Source ROI
bound – Bounding box of the transformed source ROI
aCoeffs – Perspective transform coefficients
- Returns
Error codes:
NPP_SIZE_ERROR Indicates an error condition if any image dimension has zero or negative value
NPP_RECTANGLE_ERROR Indicates an error condition if width or height of the intersection of the oSrcROI and source image is less than or equal to 1
NPP_COEFFICIENT_ERROR Indicates an error condition if coefficient values are invalid
Perspective Transform
Transforms (warps) an image based on a perspective transform.
The perspective transform is given as a \(3\times 3\) matrix C. A pixel location \((x, y)\) in the source image is mapped to the location \((x', y')\) in the destination image. The destination image coorodinates are computed as follows:
Common parameters for nppiWarpPerspective packed pixel functions:
Common parameters for nppiWarpPerspective planar pixel functions:
- param pSrc
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Perspective transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Perspective Transform Error Codes
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Perspective transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Perspective Transform Error Codes
Functions
-
NppStatus nppiWarpPerspective_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 8-bit unsigned integer perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_8u_P3R_Ctx(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_8u_P3R(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_8u_P4R_Ctx(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_8u_P4R(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 8-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer perspective warp, igoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 16-bit unsigned integer perspective warp, igoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16u_P3R_Ctx(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_16u_P3R(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_16u_P4R_Ctx(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_16u_P4R(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 16-bit unsigned integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32s_C1R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_C1R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_C3R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_C3R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_C4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_C4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_AC4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer perspective warp, igoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_AC4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit signed integer perspective warp, igoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32s_P3R_Ctx(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32s_P3R(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32s_P4R_Ctx(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32s_P4R(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 32-bit signed integer perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_16f_C1R_Ctx(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16f_C1R(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 16-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16f_C3R_Ctx(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16f_C3R(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 16-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16f_C4R_Ctx(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_16f_C4R(const Npp16f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 16-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit floating-point perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspective packed pixel functions:.
-
NppStatus nppiWarpPerspective_32f_P3R_Ctx(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32f_P3R(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32f_P4R_Ctx(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
-
NppStatus nppiWarpPerspective_32f_P4R(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 32-bit floating-point perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspective planar pixel functions:.
Perspective Transform Batch
Details of the warp perspective operation are described above in the WarpPerspective section. WarpPerspectiveBatch generally takes the same parameter list as WarpPerspective except that there is a list of N instances of those parameters (N > 1) and that list is passed in device memory. A convenient data structure is provided that allows for easy initialization of the parameter lists. The aTransformedCoeffs array is for internal use only and should not be directly initialized by the application. The only restriction on these functions is that there is one single source ROI rectangle and one single destination ROI rectangle which are applied respectively to each image in the batch. The primary purpose of this function is to provide improved performance for batches of smaller images as long as GPU resources are available. Therefore it is recommended that the function not be used for very large images as there may not be resources available for processing several large images simultaneously.
A single set of oSrcRectROI and oDstRectROI values are applied to each source image and destination image in the batch. Source and destination image sizes may vary but oSmallestSrcSize must be set to the smallest source and image size in the batch. The parameters in the
NppiWarpPerspectiveBatchCXR structure represent the corresponding per-image nppiWarpPerspective parameters for each image in the batch. The NppiWarpPerspectiveBatchCXR array must be in device memory. The nppiWarpPerspectiveBatchInit function MUST be called AFTER the application has initialized the array of NppiWarpPerspectiveBatchCXR structures and BEFORE calling any of the nppiWarpPerspectiveBatch functions to so that the aTransformedCoeffs array can be internally pre-initialized for each image in the batch. The batch size passed to nppiWarpPerspectiveBatchInit must match the batch size passed to the corresponding warp perspective batch function.WarpPerspectiveBatch supports the following interpolation modes:
NPPI_INTER_NN
NPPI_INTER_LINEAR
NPPI_INTER_CUBIC
Common parameters for nppiWarpPerspectiveBatch functions:
- param oSmallestSrcSize
Size in pixels of the entire smallest source image width and height, may be from different images.
- param oSrcRectROI
Region of interest in the source images.
- param oDstRectROI
Region of interest in the destination images.
- param eInterpolation
The type of eInterpolation to perform resampling. Currently limited to NPPI_INTER_NN, NPPI_INTER_LINEAR, or NPPI_INTER_CUBIC.
- param pBatchList
Device memory pointer to nBatchSize list of NppiWarpPerspectiveBatchCXR structures.
- param nBatchSize
Number of NppiWarpPerspectiveBatchCXR structures in this call (must be > 1).
- param nppStreamCtx
- return
Functions
-
NppStatus nppiWarpPerspectiveBatchInit_Ctx(NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
Initializes the aTransformdedCoeffs array in pBatchList for each image in the list.
MUST be called before calling the corresponding warp perspective batch function whenever any of the transformation matrices in the list have changed.
- Parameters
pBatchList – Device memory pointer to nBatchSize list of NppiWarpPerspectiveBatchCXR structures.
nBatchSize – Number of NppiWarpPerspectiveBatchCXR structures in this call (must be > 1).
nppStreamCtx – Application Managed Stream Context.
-
NppStatus nppiWarpPerspectiveBatchInit(NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
Initializes the aTransformdedCoeffs array in pBatchList for each image in the list.
MUST be called before calling the corresponding warp perspective batch function whenever any of the transformation matrices in the list have changed.
- Parameters
pBatchList – Device memory pointer to nBatchSize list of NppiWarpPerspectiveBatchCXR structures.
nBatchSize – Number of NppiWarpPerspectiveBatchCXR structures in this call (must be > 1).
-
NppStatus nppiWarpPerspectiveBatch_8u_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 8-bit unsigned integer image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 8-bit unsigned integer image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 8-bit unsigned integer image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 8-bit unsigned integer image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned integer image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 8-bit unsigned integer image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_AC4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 8-bit unsigned integer image warp perspective batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_8u_AC4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 8-bit unsigned integer image warp perspective batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_16f_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 16-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_16f_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 16-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_16f_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 16-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_16f_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 16-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_16f_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 16-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_16f_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 16-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_C1R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
1 channel 32-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_C1R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
1 channel 32-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_C3R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
3 channel 32-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_C3R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
3 channel 32-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_C4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_C4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 32-bit floating point image warp perspective batch.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_AC4R_Ctx(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize, NppStreamContext nppStreamCtx)
4 channel 32-bit floating point image warp perspective batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
-
NppStatus nppiWarpPerspectiveBatch_32f_AC4R(NppiSize oSmallestSrcSize, NppiRect oSrcRectROI, NppiRect oDstRectROI, int eInterpolation, NppiWarpPerspectiveBatchCXR *pBatchList, unsigned int nBatchSize)
4 channel 32-bit floating point image warp perspective batch not affecting alpha.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBatch functions:.
Backwards Perspective Transform
Transforms (warps) an image based on a perspective transform.
The perspective transform is given as a \(3\times 3\) matrix C. A pixel location \((x, y)\) in the source image is mapped to the location \((x', y')\) in the destination image. The destination image coorodinates fullfil the following properties:
Common parameters for nppiWarpPerspectiveBack packed pixel functions:
Common parameters for nppiWarpPerspectiveBack planar pixel functions:
- param pSrc
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Perspective transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Perspective Transform Error Codes
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nDstStep
- param oDstROI
Destination ROI.
- param aCoeffs
Perspective transform coefficients.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Perspective Transform Error Codes
Functions
-
NppStatus nppiWarpPerspectiveBack_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer backwards perspective warp, igoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 8-bit unsigned integer backwards perspective warp, igoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_P3R_Ctx(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_P3R(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_P4R_Ctx(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_8u_P4R(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 8-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer backwards perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 16-bit unsigned integer backwards perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_P3R_Ctx(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_P3R(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_P4R_Ctx(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_16u_P4R(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 16-bit unsigned integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_C1R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_C1R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_C3R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_C3R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_C4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_C4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_AC4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer backwards perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_AC4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit signed integer backwards perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_P3R_Ctx(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_P3R(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_P4R_Ctx(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32s_P4R(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel planar 32-bit signed integer backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Single-channel 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point backwards perspective warp, ignorning alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Four-channel 32-bit floating-point backwards perspective warp, ignorning alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack packed pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_P3R_Ctx(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_P3R(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_P4R_Ctx(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
-
NppStatus nppiWarpPerspectiveBack_32f_P4R(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aCoeffs[3][3], int eInterpolation)
Three-channel planar 32-bit floating-point backwards perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveBack planar pixel functions:.
Quad-Based Perspective Transform
Transforms (warps) an image based on an perspective transform.
The perspective transform is computed such that it maps a quadrilateral in source image space to a quadrilateral in destination image space.
Common parameters for nppiWarpPerspectiveQuad packed pixel functions:
Common parameters for nppiWarpPerspectiveQuad planar pixel functions:
- param pSrc
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param aSrcQuad
Source quad.
- param pDst
- param nDstStep
- param oDstROI
Destination ROI.
- param aDstQuad
Destination quad.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Perspective Transform Error Codes
- param pSrc
Source-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param oSrcSize
Size of source image in pixels.
- param nSrcStep
- param oSrcROI
Source ROI.
- param aSrcQuad
Source quad.
- param pDst
Destination-Planar-Image Pointer Array (host memory array containing device memory image plane pointers).
- param nDstStep
- param oDstROI
Destination ROI.
- param aDstQuad
Destination quad.
- param eInterpolation
Interpolation mode: can be NPPI_INTER_NN, NPPI_INTER_LINEAR or NPPI_INTER_CUBIC.
- param nppStreamCtx
- return
Image Data Related Error Codes, ROI Related Error Codes, Perspective Transform Error Codes
Functions
-
NppStatus nppiWarpPerspectiveQuad_8u_C1R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_C1R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_C3R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_C3R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_C4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_C4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_AC4R_Ctx(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 8-bit unsigned integer quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_AC4R(const Npp8u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 8-bit unsigned integer quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_P3R_Ctx(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_P3R(const Npp8u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_P4R_Ctx(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_8u_P4R(const Npp8u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp8u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 8-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_C1R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_C1R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_C3R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_C3R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_C4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_C4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_AC4R_Ctx(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 16-bit unsigned integer quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_AC4R(const Npp16u *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 16-bit unsigned integer quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_P3R_Ctx(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_P3R(const Npp16u *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_P4R_Ctx(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_16u_P4R(const Npp16u *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp16u *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 16-bit unsigned integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_C1R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_C1R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_C3R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_C3R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_C4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_C4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_AC4R_Ctx(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit signed integer quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_AC4R(const Npp32s *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit signed integer quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_P3R_Ctx(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_P3R(const Npp32s *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_P4R_Ctx(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32s_P4R(const Npp32s *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32s *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 32-bit signed integer quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_C1R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Single-channel 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_C1R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Single-channel 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_C3R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_C3R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_C4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_C4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_AC4R_Ctx(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel 32-bit floating-point quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_AC4R(const Npp32f *pSrc, NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst, int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel 32-bit floating-point quad-based perspective warp, ignoring alpha channel.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad packed pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_P3R_Ctx(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Three-channel planar 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_P3R(const Npp32f *pSrc[3], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[3], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Three-channel planar 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_P4R_Ctx(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation, NppStreamContext nppStreamCtx)
Four-channel planar 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.
-
NppStatus nppiWarpPerspectiveQuad_32f_P4R(const Npp32f *pSrc[4], NppiSize oSrcSize, int nSrcStep, NppiRect oSrcROI, const double aSrcQuad[4][2], Npp32f *pDst[4], int nDstStep, NppiRect oDstROI, const double aDstQuad[4][2], int eInterpolation)
Four-channel planar 32-bit floating-point quad-based perspective warp.
For common parameter descriptions, see Common parameters for nppiWarpPerspectiveQuad planar pixel functions:.