Overview
The Image Resampling application resamples the input image by first applying a low-pass filter to avoid aliasing, then doing a downsampling. The resulting image has half of input's width and one third of input's height. The result is then saved to disk.
This sample shows the following:
- Creating and destroying a VPI stream.
- Wrapping an image hosted on CPU (the input) to be used by VPI
- Creating a VPI-managed 2D image.
- Create a pipeline with two operations, GaussianFilter and Resample.
- Simple stream synchronization.
- Image locking to access its contents from CPU side.
- Error handling.
- Environment clean up.
Instructions
The usage is:
./vpi_sample_04_resample <backend> <input image>
where
- backend: either cpu, cuda or pva; it defines the backend that will perform the processing.
- input image: input image file name to be downsampled, it accepts png, jpeg and possibly others.
Here's one example:
./vpi_sample_04_resample cuda ../assets/kodim8.png
This is using the CUDA backend and one of the provided sample images. You can try with other images, respecting the constraints imposed by the algorithm.
Results
Input image | Output image, downsampled |
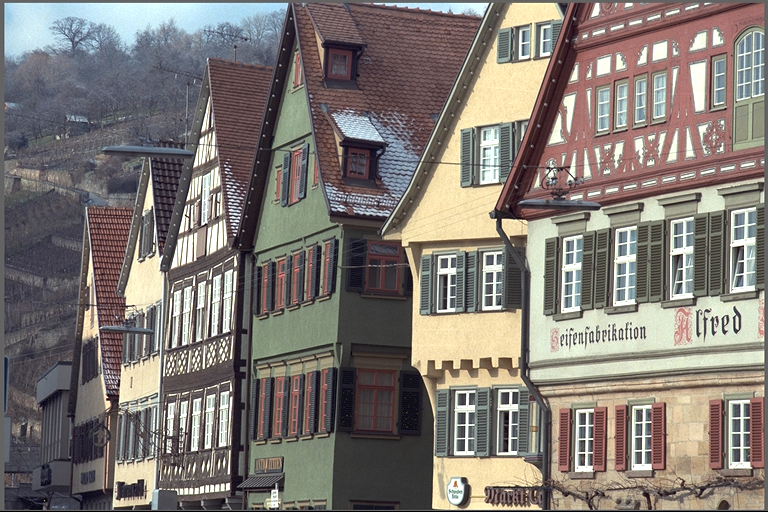 | |
Source code
For convenience, here's the code that is also installed in the samples directory.
#include <opencv2/core/version.hpp>
#if CV_MAJOR_VERSION >= 3
# include <opencv2/imgcodecs.hpp>
#else
# include <opencv2/highgui/highgui.hpp>
#endif
#include <cstring>
#include <iostream>
#define CHECK_STATUS(STMT) \
do \
{ \
VPIStatus status = (STMT); \
if (status != VPI_SUCCESS) \
{ \
throw std::runtime_error(vpiStatusGetName(status)); \
} \
} while (0);
int main(int argc, char *argv[])
{
int retval = 0;
try
{
if (argc != 3)
{
throw std::runtime_error(std::string("Usage: ") + argv[0] + " <cpu|pva|cuda> <input image>");
}
std::string strDevType = argv[1];
std::string strInputFileName = argv[2];
cv::Mat cvImage = cv::imread(strInputFileName, cv::IMREAD_GRAYSCALE);
if (cvImage.empty())
{
throw std::runtime_error("Can't open '" + strInputFileName + "'");
}
assert(cvImage.type() == CV_8UC1);
if (strDevType == "cpu")
{
}
else if (strDevType == "cuda")
{
}
else if (strDevType == "pva")
{
}
else
{
throw std::runtime_error("Backend '" + strDevType +
"' not recognized, it must be either cpu, cuda or pva.");
}
{
memset(&imgData, 0, sizeof(imgData));
}
{
imwrite("resampled_" + strDevType + ".png", cvOut);
}
}
catch (std::exception &e)
{
std::cerr << e.what() << std::endl;
retval = 1;
}
if (stream != NULL)
{
}
return retval;
}