|
VPI - Vision Programming Interface
0.4.4 Release
|
Overview
The Rescale application rescales the input image by first applying a low-pass filter to avoid aliasing, then doing a downsampling. The resulting image has half of input's width and one third of input's height. The result is then saved to disk.
This sample shows the following:
- Creating and destroying a VPI stream.
- Wrapping an image hosted on CPU (the input) to be used by VPI
- Creating a VPI-managed 2D image.
- Create a pipeline with two operations, GaussianFilter and Rescale.
- Simple stream synchronization.
- Image locking to access its contents from CPU side.
- Error handling.
- Environment clean up.
Instructions
The usage is:
./vpi_sample_04_rescale <backend> <input image>
where
- backend: either cpu, cuda or pva; it defines the backend that will perform the processing.
- input image: input image file name to be downsampled, it accepts png, jpeg and possibly others.
Here's one example:
./vpi_sample_04_rescale cuda ../assets/kodim8.png
This is using the CUDA backend and one of the provided sample images. You can try with other images, respecting the constraints imposed by the algorithm.
Results
Input image | Output image, downsampled |
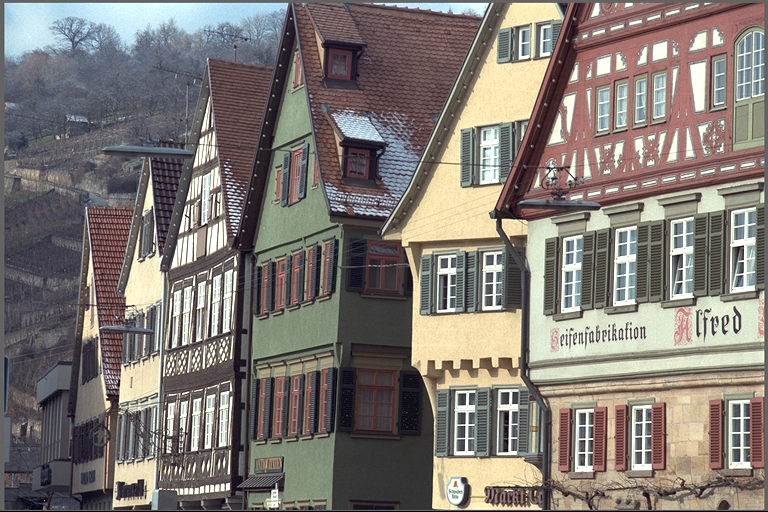 | |
Source code
For convenience, here's the code that is also installed in the samples directory.
29 #include <opencv2/core/version.hpp>
30 #if CV_MAJOR_VERSION >= 3
31 # include <opencv2/imgcodecs.hpp>
33 # include <opencv2/highgui/highgui.hpp>
46 #define CHECK_STATUS(STMT) \
49 VPIStatus status = (STMT); \
50 if (status != VPI_SUCCESS) \
52 char buffer[VPI_MAX_STATUS_MESSAGE_LENGTH]; \
53 vpiGetLastStatusMessage(buffer, sizeof(buffer)); \
54 std::ostringstream ss; \
55 ss << vpiStatusGetName(status) << ": " << buffer; \
56 throw std::runtime_error(ss.str()); \
60 int main(
int argc,
char *argv[])
74 throw std::runtime_error(std::string(
"Usage: ") + argv[0] +
" <cpu|vic|cuda> <input image>");
77 std::string strBackend = argv[1];
78 std::string strInputFileName = argv[2];
81 cv::Mat cvImage = cv::imread(strInputFileName);
84 throw std::runtime_error(
"Can't open '" + strInputFileName +
"'");
87 assert(cvImage.type() == CV_8UC3);
92 if (strBackend ==
"cpu")
96 else if (strBackend ==
"cuda")
100 else if (strBackend ==
"vic")
106 throw std::runtime_error(
"Backend '" + strBackend +
"' not recognized, it must be either cpu, cuda or vic");
118 memset(&imgData, 0,
sizeof(imgData));
168 imwrite(
"scaled_" + strBackend +
".png", cvOut);
174 catch (std::exception &e)
176 std::cerr << e.what() << std::endl;
uint32_t height
Height of this plane in pixels.
uint32_t width
Width of this plane in pixels.
VPIStatus vpiStreamCreate(uint32_t flags, VPIStream *stream)
Create a stream instance.
VPIBackend
VPI Backend types.
@ VPI_LOCK_READ
Lock memory only for reading.
VPIStatus vpiImageUnlock(VPIImage img)
Releases the lock on an image object.
VPIStatus vpiStreamSync(VPIStream stream)
Blocks the calling thread until all submitted commands in this stream queue are done (queue is empty)...
@ VPI_BACKEND_CUDA
CUDA backend.
Stores information about image characteristics and content.
struct VPIStreamImpl * VPIStream
A handle to a stream.
Declares functions that implement the Rescale algorithm.
@ VPI_INTERP_LINEAR
Alias to fast linear interpolation.
void vpiStreamDestroy(VPIStream stream)
Destroy a stream instance and deallocate all HW resources.
VPIImagePlane planes[VPI_MAX_PLANE_COUNT]
Data of all image planes.
VPIStatus vpiImageCreate(uint32_t width, uint32_t height, VPIImageFormat fmt, uint32_t flags, VPIImage *img)
Create an empty image instance with the specified flags.
void vpiImageDestroy(VPIImage img)
Destroy an image instance.
Functions and structures for dealing with VPI images.
uint32_t pitchBytes
Difference in bytes of beginning of one row and the beginning of the previous.
struct VPIImageImpl * VPIImage
A handle to an image.
int32_t numPlanes
Number of planes.
VPIStatus vpiSubmitRescale(VPIStream stream, VPIBackend backend, VPIImage input, VPIImage output, VPIInterpolationType interpolationType, VPIBoundaryCond boundary)
Changes the size and scale of a 2D image.
@ VPI_BACKEND_VIC
VIC backend.
VPIStatus vpiImageLock(VPIImage img, VPILockMode mode, VPIImageData *hostData)
Acquires the lock on an image object and returns a pointer to the image planes.
VPIImageFormat type
Image type.
@ VPI_BOUNDARY_COND_CLAMP
Border pixels are repeated indefinitely.
Declaration of VPI status codes handling functions.
@ VPI_BACKEND_CPU
CPU backend.
VPIStatus vpiImageCreateHostMemWrapper(const VPIImageData *hostData, uint32_t flags, VPIImage *img)
Create an image object by wrapping around an existing host memory block.
Declares functions dealing with VPI streams.
void * data
Pointer to the first row of this plane.