Core Concepts
Before we discuss the details of the graph and legacy APIs, this section introduces the key concepts that are common to both.
cuDNN Handle
The cuDNN library exposes a host API but assumes that for operations using the GPU, the necessary data is directly accessible from the device.
An application using cuDNN must initialize a handle to the library context by calling cudnnCreate(). This handle is explicitly passed to every subsequent library function that operates on GPU data. Once the application finishes using cuDNN, it can release the resources associated with the library handle using cudnnDestroy(). This approach allows the user to explicitly control the library’s functioning when using multiple host threads, GPUs, and CUDA streams.
For example, an application can use cudaSetDevice (prior to creating a cuDNN handle) to associate different devices with different host threads, and in each of those host threads, create a unique cuDNN handle that directs the subsequent library calls to the device associated with it. In this case, the cuDNN library calls made with different handles would automatically run on different devices.
The device associated with a particular cuDNN context is assumed to remain unchanged between the corresponding cudnnCreate() and cudnnDestroy() calls. In order for the cuDNN library to use a different device within the same host thread, the application must set the new device to be used by calling cudaSetDevice
and then create another cuDNN context, which will be associated with the new device, by calling cudnnCreate().
Tensors and Layouts
Whether using the graph API or the legacy API, cuDNN operations take tensors as input and produce tensors as output.
Tensor Descriptor
The cuDNN library describes data with a generic n-D tensor descriptor defined with the following parameters:
a number of dimensions from 3 to 8
a data type (32-bit floating-point, 64 bit-floating point, 16-bit floating-point…)
an integer array defining the size of each dimension
an integer array defining the stride of each dimension (for example, the number of elements to add to reach the next element from the same dimension)
This tensor definition allows, for example, to have some dimensions overlapping each other within the same tensor by having the stride of one dimension smaller than the product of the dimension and the stride of the next dimension. In cuDNN, unless specified otherwise, all routines will support tensors with overlapping dimensions for forward-pass input tensors, however, dimensions of the output tensors cannot overlap. Even though this tensor format supports negative strides (which can be useful for data mirroring), cuDNN routines do not support tensors with negative strides unless specified otherwise.
WXYZ Tensor Descriptor
Tensor descriptor formats are identified using acronyms, with each letter referencing a corresponding dimension. In this document, the usage of this terminology implies:
all the strides are strictly positive
the dimensions referenced by the letters are sorted in decreasing order of their respective strides
3-D Tensor Descriptor
A 3-D tensor is commonly used for matrix multiplications, with three letters: B, M, and N. B represents the batch size (for batch GEMM, set to 1 for single GEMM), M represents the number of rows, and N represents the number of columns. Refer to the CUDNN_BACKEND_OPERATION_MATMUL_DESCRIPTOR operation for more information.
4-D Tensor Descriptor
A 4-D tensor descriptor is used to define the format for batches of 2D images with 4 letters: N,C,H,W for respectively the batch size, the number of feature maps, the height and the width. The letters are sorted in decreasing order of the strides. The commonly used 4-D tensor formats are:
NCHW
NHWC
CHWN
5-D Tensor Descriptor
A 5-D tensor descriptor is used to define the format of the batch of 3D images with 5 letters: N,C,D,H,W for respectively the batch size, the number of feature maps, the depth, the height, and the width. The letters are sorted in decreasing order of the strides. The commonly used 5-D tensor formats are called:
NCDHW
NDHWC
CDHWN
Fully-Packed Tensors
A tensor is defined as XYZ-fully-packed
if, and only if:
the number of tensor dimensions is equal to the number of letters preceding the
fully-packed
suffixthe stride of the i-th dimension is equal to the product of the (i+1)-th dimension by the (i+1)-th stride
the stride of the last dimension is 1
Partially-Packed Tensors
The partially XYZ-packed
terminology only applies in the context of a tensor format described with a superset of the letters used to define a partially-packed tensor. A WXYZ tensor is defined as XYZ-packed
if, and only if:
the strides of all dimensions NOT referenced in the
-packed
suffix are greater or equal to the product of the next dimension by the next stride.the stride of each dimension referenced in the
-packed
suffix in position i is equal to the product of the (i+1)-st dimension by the (i+1)-st stride.if the last tensor’s dimension is present in the
-packed
suffix, its stride is 1.
For example, an NHWC tensor WC-packed
means that the c_stride
is equal to 1 and w_stride
is equal to c_dim x c_stride
. In practice, the -packed
suffix is usually applied to the minor dimensions of a tensor but can be applied to only the major dimensions; for example, an NCHW tensor that is only N-packed
.
Spatially Packed Tensors
Spatially-packed tensors are defined as partially-packed in spatial dimensions. For example, a spatially-packed 4D tensor would mean that the tensor is either NCHW HW-packed or CNHW HW-packed.
Overlapping Tensors
A tensor is defined to be overlapping if iterating over a full range of dimensions produces the same address more than once. In practice an overlapped tensor will have stride[i-1] < stride[i]*dim[i]
for some of the i
from [1,nbDims]
interval.
Data Layout Formats
This section describes how cuDNN tensors are arranged in memory according to several data layout formats.
The recommended way to specify the layout format of a tensor is by setting its strides accordingly. For compatibility with the v7 API, a subset of the layout formats can also be configured through the cudnnTensorFormat_t enum. The enum is only supplied for legacy reasons and is deprecated.
Example Tensor
Consider a batch of images with the following dimensions:
N is the batch size; 1
C is the number of feature maps (that is, number of channels); 64
H is the image height; 5
W is the image width; 4
To keep the example simple, the image pixel elements are expressed as a sequence of integers, 0, 1, 2, 3, and so on.
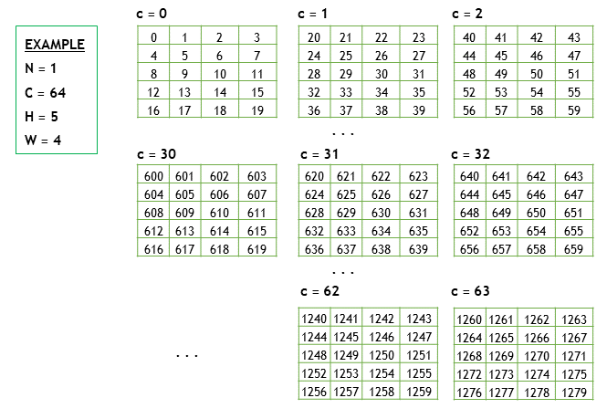
In the following subsections, we’ll use the above example to demonstrate the different layout formats.
Convolution Layouts
cuDNN supports several layouts for convolution, as described in the following sections.
NCHW Memory Layout
The above 4D tensor is laid out in the memory in the NCHW format)as below:
Beginning with the first channel (c=0), the elements are arranged contiguously in row-major order.
Continue with second and subsequent channels until the elements of all the channels are laid out.
Proceed to the next batch (if N is > 1).
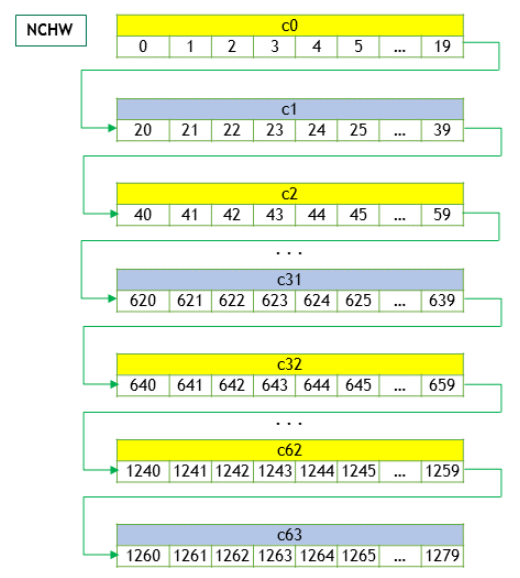
NHWC Memory Layout
For the NHWC memory layout, the corresponding elements in all the C channels are laid out first, as below:
Begin with the first element of channel 0, then proceed to the first element of channel 1, and so on, until the first elements of all the C channels are laid out.
Next, select the second element of channel 0, then proceed to the second element of channel 1, and so on, until the second element of all the channels are laid out.
Follow the row-major order of channel 0 and complete all the elements.
Proceed to the next batch (if N is > 1).
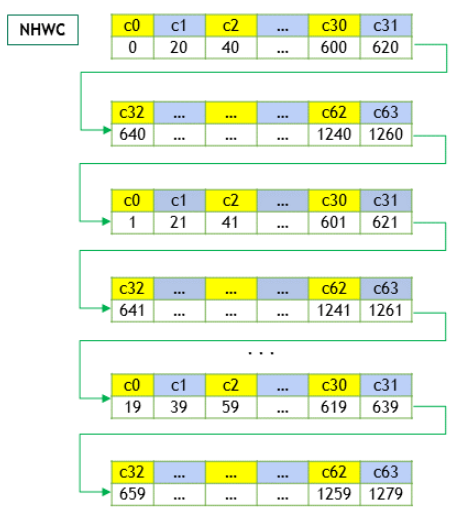
NC/32HW32 Memory Layout
The NC/32HW32 is similar to NHWC, with a key difference. For the NC/32HW32 memory layout, the 64 channels are grouped into two groups of 32 channels each - first group consisting of channels c0
through c31
, and the second group consisting of channels c32
through c63
. Then each group is laid out using the NHWC format.
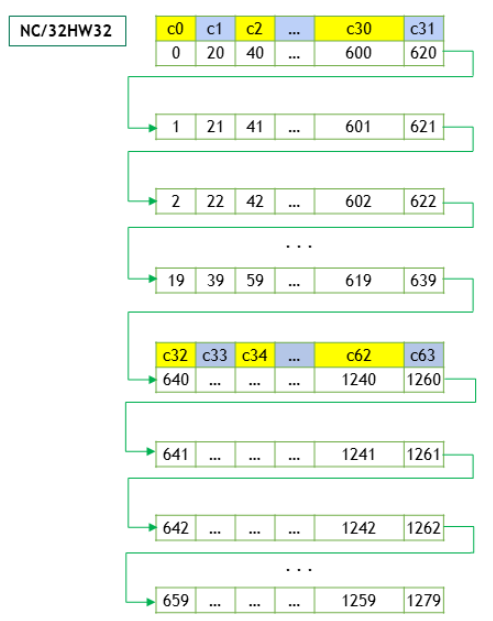
For the generalized NC/xHWx layout format, the following observations apply:
Only the channel dimension,
C
, is grouped intox
channels each.When
x = 1
, each group has only one channel. Hence, the elements of one channel (that is, one group) are arranged contiguously (in the row-major order), before proceeding to the next group (that is, next channel). This is the same as the NCHW format.When
x = C
, then NC/xHWx is identical to NHWC, that is, the entire channel depthC
is considered as a single group. The casex = C
can be thought of as vectorizing the entireC
dimension as one big vector, laying out all theC
, followed by the remaining dimensions, just like NHWC.The tensor format cudnnTensorFormat_t can also be interpreted in the following way - The NCHW INT8x32 format is really
N x (C/32) x H x W x 32
(32C``s for every ``W
), just as the NCHW INT8x4 format isN x (C/4) x H x W x 4
(4C
for everyW
). Hence theVECT_C
name - eachW
is a vector (4 or 32) ofC
.
MatMul Layouts
As discussed in 3-D Tensor Descriptor, matmul uses 3D tensors, described using BMN dimensions. The layout can be specified through the following strides. The following are two examples of recommended layouts:
Packed Row-major: dim [B,M,N] with stride [MN, N, 1], or
Packed Column-major: dim [B,M,N] with stride [MN, 1, M]
Unpacked layouts for 3-D tensors are supported as well, but their support surface is more ragged.
Tensor Core Operations
The cuDNN v7 library introduced the acceleration of compute-intensive routines using Tensor Core hardware on supported GPU SM versions. Tensor Core operations are supported beginning with the NVIDIA Volta GPU.
Tensor Core operations accelerate matrix math operations; cuDNN uses Tensor Core operations that accumulate into FP16, FP32, and INT32 values. Setting the math mode to CUDNN_TENSOR_OP_MATH
via the cudnnMathType_t enumerator indicates that the library will use Tensor Core operations. This enumerator specifies the available options to enable the Tensor Core and should be applied on a per-routine basis.
The default math mode is CUDNN_DEFAULT_MATH
, which indicates that the Tensor Core operations will be avoided by the library. Because the CUDNN_TENSOR_OP_MATH
mode uses the Tensor Cores, it is possible that these two modes generate slightly different numerical results due to different sequencing of the floating-point operations.
For example, the result of multiplying two matrices using Tensor Core operations is very close, but not always identical, to the result achieved using a sequence of scalar floating-point operations. For this reason, the cuDNN library requires an explicit user opt-in before enabling the use of Tensor Core operations.
However, experiments with training common deep learning models show negligible differences between using Tensor Core operations and scalar floating point paths, as measured by both the final network accuracy and the iteration count to convergence. Consequently, the cuDNN library treats both modes of operation as functionally indistinguishable and allows for the scalar paths to serve as legitimate fallbacks for cases in which the use of Tensor Core operations is unsuitable.
Kernels using Tensor Core operations are available for:
Convolutions
RNNs
Multihead Attention
For more information, refer to NVIDIA Training with Mixed Precision.
For a deep learning compiler, the following are the key guidelines:
Make sure that the convolution operation is eligible for Tensor Cores by avoiding any combinations of large padding and large filters.
Transform the inputs and filters to NHWC, pre-pad channel and batch size to be a multiple of 8.
Make sure that all user-provided tensors, workspace, and reserve space are aligned to 128-bit boundaries. Note that 1024-bit alignment may deliver better performance.
Notes on Tensor Core Precision
For FP16 data, Tensor Cores operate on FP16 input, output in FP16, and may accumulate in FP16 or FP32. The FP16 multiply leads to a full-precision result that is accumulated in FP32 operations with the other products in a given dot product for a matrix with m x n x k
dimensions.
For an FP32 accumulation, with FP16 output, the output of the accumulator is down-converted to FP16. Generally, the accumulation type is of greater or equal precision to the output type.
