Augmentation GalleryΒΆ
This example showcases different augmentations possible with DALI.
In [1]:
from nvidia.dali.pipeline import Pipeline
import nvidia.dali.ops as ops
import nvidia.dali.types as types
import numpy as np
from timeit import default_timer as timer
import numpy as np
import matplotlib.pyplot as plt
db_folder = "/data/imagenet/train-lmdb-256x256"
class HybridPipe(Pipeline):
def __init__(self, batch_size, num_threads, device_id, pipelined = True, async = True):
super(HybridPipe, self).__init__(batch_size, num_threads, device_id, seed = 12, exec_pipelined=pipelined, exec_async=async)
self.input = ops.CaffeReader(path = db_folder, random_shuffle = True)
self.decode = ops.nvJPEGDecoder(device = "mixed", output_type = types.RGB)
self.cmnp = ops.CropMirrorNormalize(device = "gpu",
output_dtype = types.FLOAT,
output_layout = types.NHWC,
crop = (224, 224),
image_type = types.RGB,
mean = [0., 0., 0.],
std = [1., 1., 1.])
self.augmentations = []
self.augmentations.append(ops.Jitter(device = "gpu"))
self.augmentations.append(ops.Water(device = "gpu"))
self.augmentations.append(ops.Sphere(device = "gpu"))
self.augmentations.append(ops.Rotate(device = "gpu", angle = 30.0, interp_type = types.INTERP_LINEAR))
self.augmentations.append(ops.WarpAffine(device = "gpu", matrix = [1.0, 0.8, 0.0, 0.0, 1.2, 0.0],
use_image_center = True, interp_type = types.INTERP_LINEAR))
self.augmentations.append(ops.Contrast(device = "gpu", contrast = 1.5))
self.augmentations.append(ops.Brightness(device = "gpu", brightness = 0.5))
self.augmentations.append(ops.Saturation(device = "gpu", saturation = 0.2))
self.augmentations.append(ops.Hue(device = "gpu", hue = 45.))
self.uniform = ops.Uniform(range = (0.0, 1.0))
self.iter = 0
def define_graph(self):
self.jpegs, self.labels = self.input()
images = self.decode(self.jpegs)
n = len(self.augmentations)
outputs = [images for _ in range(n+1)]
# outputs[0] is the original cropped image
for i in range(n):
outputs[i+1] = self.augmentations[i](outputs[i+1])
cropped_images = self.cmnp(outputs,
crop_pos_x = self.uniform(),
crop_pos_y = self.uniform())
return [self.labels] + cropped_images
def iter_setup(self):
pass
In [2]:
batch_size = 32
pipe = HybridPipe(batch_size=batch_size, num_threads=2, device_id = 0)
pipe.build()
In [3]:
pipe_out = pipe.run()
In [4]:
n = 5
from synsets import imagenet_synsets
import matplotlib.gridspec as gridspec
len_outputs = len(pipe_out) - 1
augmentations = ["original",
"jitter",
"water",
"sphere",
"rotate",
"warpaffine",
"contrast",
"brightness",
"saturation",
"hue"]
fig = plt.figure(figsize = (16,16))
plt.suptitle(imagenet_synsets[pipe_out[0].at(n)[0]], fontsize=16)
columns = 4
rows = 4
gs = gridspec.GridSpec(rows, columns)
for i in range(len_outputs):
plt.subplot(gs[i])
plt.axis("off")
plt.title(augmentations[i])
pipe_out_cpu = pipe_out[1 + i].asCPU()
img_chw = pipe_out_cpu.at(n)
plt.imshow((img_chw)/255.0)
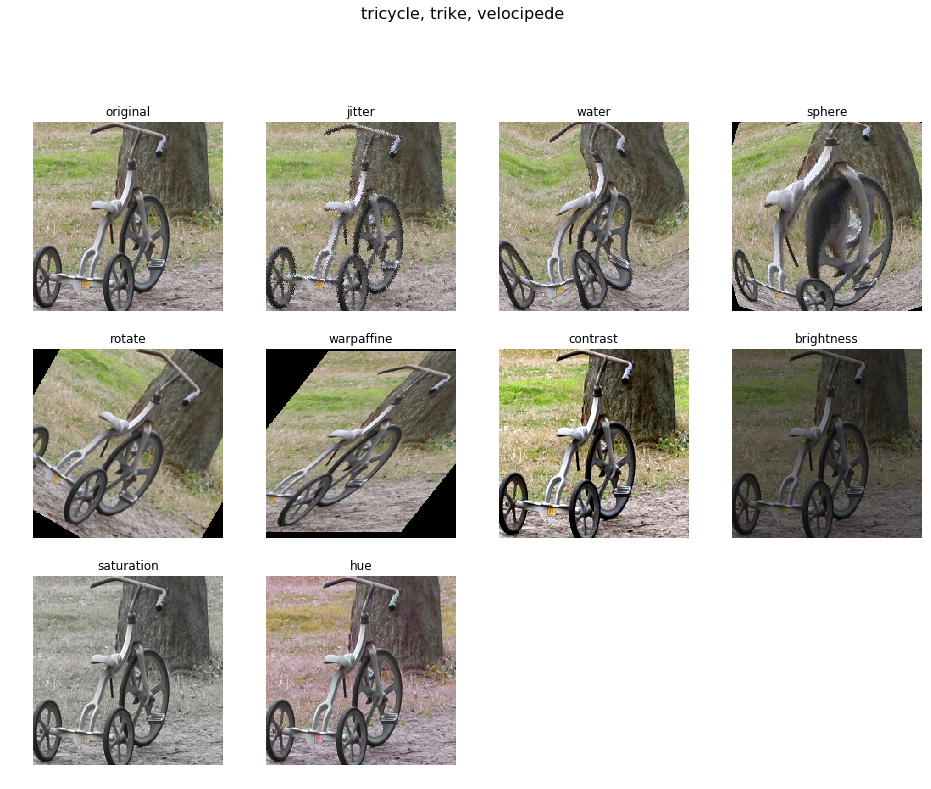