Overview
This sample shows how interoperability between VPI and PyTorch works in Python. It shows a simple set of image processing operations applied on a sample input image in Pytorch and VPI without any memory copies during the interoperation.
This sample uses PyTorch as an example, however, this interoperability is possible with any library that supports the cuda array interface.
Libraries such as:
The sample starts with an image given by you, converted to grayscale, then performs the following sequence of operations to it:
- Lower the image intensity using PyTorch
- Does PyTorch -> VPI interoperability without memory copies
vpi_image = vpi.asimage(torch_image)
- Applies a box filter using VPI
- Does VPI -> PyTorch interoperability without memory copies
with vpi_image.rlock_cuda() as cuda_buffer:
torch_image = torch.as_tensor(cuda_buffer)
or, with an additional deep memory copy torch_image = torch.as_tensor(vpi_image.cuda())
- Increase the image intensity using PyTorch
The result is then saved to disk.
Instructions
The usage is:
python3 main.py <input image>
where
- input image: input image file name; it accepts png, jpeg and possibly others.
Here's one example:
- Python
python3 main.py ../assets/kodim08.png
The sample will produce vpi_pytorch.png
as output file.
Results
Input image | Output image |
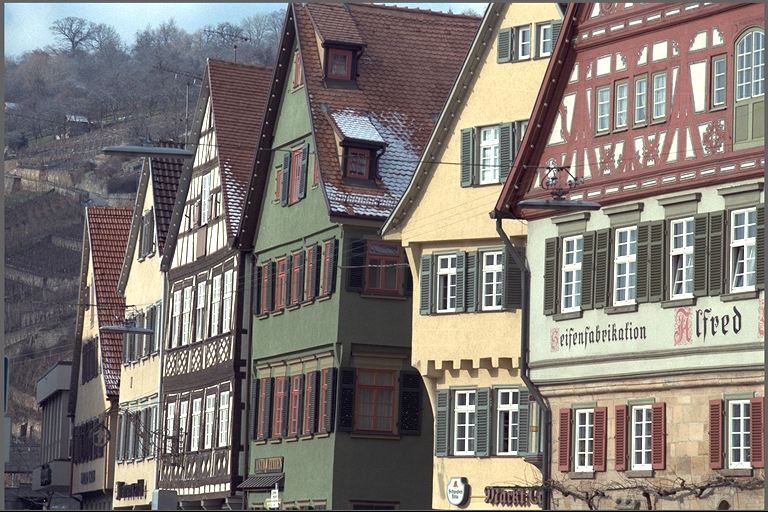 | |
Source Code
For convenience, here's the code that is also installed in the samples directory.
31 from PIL
import Image, ImageOps
32 from argparse
import ArgumentParser
35 parser = ArgumentParser()
36 parser.add_argument(
'input', help=
'Image to be used as input')
38 args = parser.parse_args()
41 assert torch.cuda.is_available()
42 cuda_device = torch.device(
'cuda')
46 pil_image = ImageOps.grayscale(Image.open(args.input))
48 sys.exit(
"Input file not found")
50 sys.exit(
"Error with input file")
53 np_image = np.asarray(pil_image)
56 torch_image = torch.asarray(np_image).cuda()
59 torch_image = torch_image/255 * 0.5
62 vpi_image = vpi.asimage(torch_image)
65 with vpi.Backend.CUDA:
67 vpi_image = vpi_image.box_filter(3)
70 with vpi_image.rlock_cuda()
as cuda_buffer:
72 torch_tensor = torch.as_tensor(cuda_buffer, device=cuda_device)
73 torch_image = torch_tensor*255 + 128
76 np_image = np.asarray(torch_image.cpu())
79 pil_image = Image.fromarray(np_image)
82 pil_image.convert(
'L').save(
'vpi_pytorch.png')