NvUIGraphic Class Reference
A graphical element, with no interactivity. More...
#include <NvUI.h>
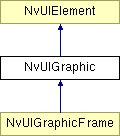
Public Member Functions | |
NvUIGraphic (const std::string &texname, float dstw=0, float dsth=0) | |
Basic constructor, taking texture filename as source. | |
NvUIGraphic (uint32_t texId, bool alpha, uint32_t srcw, uint32_t srch, float dstw=0, float dsth=0) | |
Basic constructor, taking GL texture ID as source. | |
NvUIGraphic (NvUITexture *tex, float dstw=0, float dsth=0) | |
Basic constructor, taking NvUITexture object as source. | |
virtual bool | LoadTexture (const std::string &texname, bool resetDimensions=true) |
Loads a texture from cache or file. | |
void | SetTexture (NvUITexture *tex) |
Change this graphic to use a specific NvUITexture object. | |
void | SetTextureID (const uint32_t texID, bool alpha, uint32_t srcw, uint32_t srch) |
Change this graphic to use an existing GL texture ID and details. | |
void | SetTextureFiltering (uint32_t minFilter, uint32_t magFilter) |
Helper method to set the GL texture filtering on our texture object. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Does the heavy lifting to render our texture at target position/dimensions. | |
virtual void | SetColor (NvPackedColor color) |
Sets a color value to multiply with during fragment processing. | |
void | FlushTexture () |
If we have a texture object, deletes our reference, and nulls out our member. | |
uint32_t | GetTexWidth () |
Accessor to get our texture's width. | |
uint32_t | GetTexHeight () |
Accessor to get our texture's height. | |
int32_t | GetTexID () |
Accessor to get our texture's GL texture object ID. | |
void | FlipVertical (bool flipped=true) |
Set whether to vertically-flip our texture during Draw method. | |
Protected Attributes | |
NvUITexture * | m_tex |
The texture we render from. | |
bool | m_scale |
Are we scaling the texture (pixel size other than original width/height texel size)? | |
bool | m_vFlip |
Do we need to vertical flip the texture/graphic? | |
NvPackedColor | m_color |
An RGB color to modulate with the texels of the graphic (alpha is ignored for the moment). |
Detailed Description
A graphical element, with no interactivity.This class renders a texture (or portion of one) to the screen, but has no interactivity with the user, just the visual. Other UI classes derive from this functionality, or otherwise utilize or proxy to it.
NvUIGraphic can be initialized from a texture on disk, an existing NvUITexture object, or an existing GL texture ID.
Constructor & Destructor Documentation
NvUIGraphic::NvUIGraphic | ( | const std::string & | texname, | |
float | dstw = 0 , |
|||
float | dsth = 0 | |||
) |
Basic constructor, taking texture filename as source.
Note it uses the texture size for target w/h unless default params overridden, in which case it will scale the texture appropriately.
- Parameters:
-
texname Texture filename (and path if any needed) in a std::string. dstw Target width dimension, defaults to texture width if not overridden. dsth Target height dimension, defaults to texture height if not overridden.
NvUIGraphic::NvUIGraphic | ( | uint32_t | texId, | |
bool | alpha, | |||
uint32_t | srcw, | |||
uint32_t | srch, | |||
float | dstw = 0 , |
|||
float | dsth = 0 | |||
) |
Basic constructor, taking GL texture ID as source.
Note it uses the texture size for target w/h unless default params overridden, in which case it will scale the texture appropriately.
- Parameters:
-
texId GL texture object ID. alpha Whether the source texture format contains an alpha channel. srcw Source texture width in texels. srch Source texture height in texels. dstw Target width dimension, defaults to texture width if not overridden. dsth Target height dimension, defaults to texture height if not overridden.
NvUIGraphic::NvUIGraphic | ( | NvUITexture * | tex, | |
float | dstw = 0 , |
|||
float | dsth = 0 | |||
) |
Basic constructor, taking NvUITexture object as source.
Note it uses the texture size for target w/h unless default params overridden, in which case it will scale the texture appropriately.
- Parameters:
-
tex Source NvUITexture object pointer. dstw Target width dimension, defaults to texture width if not overridden. dsth Target height dimension, defaults to texture height if not overridden.
Member Function Documentation
virtual void NvUIGraphic::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
Does the heavy lifting to render our texture at target position/dimensions.
Reimplemented from NvUIElement.
Reimplemented in NvUIGraphicFrame.
void NvUIGraphic::FlipVertical | ( | bool | flipped = true |
) | [inline] |
Set whether to vertically-flip our texture during Draw method.
void NvUIGraphic::FlushTexture | ( | ) |
If we have a texture object, deletes our reference, and nulls out our member.
uint32_t NvUIGraphic::GetTexHeight | ( | ) | [inline] |
Accessor to get our texture's height.
int32_t NvUIGraphic::GetTexID | ( | ) | [inline] |
Accessor to get our texture's GL texture object ID.
uint32_t NvUIGraphic::GetTexWidth | ( | ) | [inline] |
Accessor to get our texture's width.
virtual bool NvUIGraphic::LoadTexture | ( | const std::string & | texname, | |
bool | resetDimensions = true | |||
) | [virtual] |
Loads a texture from cache or file.
This actually ends up loading data using the NvUITexture::CacheTexture method, to take advantage of the texture cache, and loaded from disk into the cache if not already there.
- Parameters:
-
texname Filename of texture to load resetDimensions Whether to set our dimensions to match those of the texture. Defaults true.
Reimplemented in NvUIGraphicFrame.
virtual void NvUIGraphic::SetColor | ( | NvPackedColor | color | ) | [virtual] |
Sets a color value to multiply with during fragment processing.
Setting to white (1,1,1,x) color effectively disables colorization.
void NvUIGraphic::SetTexture | ( | NvUITexture * | tex | ) |
Change this graphic to use a specific NvUITexture object.
void NvUIGraphic::SetTextureFiltering | ( | uint32_t | minFilter, | |
uint32_t | magFilter | |||
) |
Helper method to set the GL texture filtering on our texture object.
void NvUIGraphic::SetTextureID | ( | const uint32_t | texID, | |
bool | alpha, | |||
uint32_t | srcw, | |||
uint32_t | srch | |||
) |
Change this graphic to use an existing GL texture ID and details.
Member Data Documentation
NvUITexture* NvUIGraphic::m_tex [protected] |
The texture we render from.
The documentation for this class was generated from the following file: