NvUIProxy Class Reference
A UI element that 'proxies' calls to another element. More...
#include <NvUI.h>
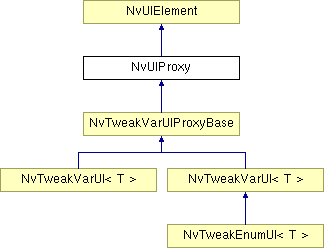
Public Member Functions | |
NvUIProxy (NvUIElement *el) | |
virtual void | Draw (const NvUIDrawState &drawState) |
Pure virtual rendering method for widgets. | |
virtual NvUIEventResponse | HandleEvent (const NvGestureEvent &ev, NvUST timeUST, NvUIElement *hasFocus) |
Virtual user interaction handling method. | |
virtual NvUIEventResponse | HandleReaction (const NvUIReaction &react) |
Virtual method for handling NvUIReaction in response to user interaction. | |
virtual void | SetOrigin (float x, float y) |
Virtual method for setting 2D origin point in UI/view space. | |
virtual void | SetDimensions (float w, float h) |
Virtual method for setting the dimensions of this element in pixels. | |
virtual void | SetDepth (float z) |
Accessor to set the Z/depth value of our UI rectangle. | |
virtual bool | HasDepth () |
Method to test whether the element's bounding rect has a non-zero Z/depth value. | |
virtual void | GetScreenRect (NvUIRect &rect) |
Accessor to retrieve the UI-space NvUIRect for this element into a passed-in reference. | |
virtual float | GetWidth () |
Accessor to get the width of this element's bounding rect. | |
virtual float | GetHeight () |
Accessor to get the height of this element's bounding rect. | |
virtual void | SetVisibility (bool show) |
Set whether or not this element is visible and thus to be drawn. | |
virtual bool | GetVisibility () |
Get whether or not this element is visible and thus to be drawn. | |
virtual void | SetAlpha (float a) |
Set the alpha-blend amount for this element. | |
virtual float | GetAlpha () |
Get the current alpha-blend override level for this element. | |
virtual void | GotFocus () |
Virtual method for telling this element it has interaction-focus (was pressed, was hit). | |
virtual void | LostFocus () |
Virtual method for telling this element it has lost interaction-focus. | |
virtual uint32_t | GetSlideFocusGroup () |
Get the SlideFocusGroup identifier for this element. | |
uint32_t | GetUID () |
While we DO have a UID for ourselves, we always return the UID of the proxy instead. | |
virtual bool | Hit (float x, float y) |
Hit-test a given point against this element's UI rectangle, using no extra margin. | |
virtual void | SetDrawState (uint32_t n) |
Set the current drawing 'state' or index. |
Detailed Description
A UI element that 'proxies' calls to another element.This is used to create further specialized subclasses of NvUIElement that have an existing type of widget they want to use for their visual and iteractive methods, but can't subclass as it could be ANY class and isn't known up front which ones would be used.
Member Function Documentation
virtual void NvUIProxy::Draw | ( | const NvUIDrawState & | drawState | ) | [inline, virtual] |
Pure virtual rendering method for widgets.
Pure virtual as there is no base implementation, it must be implemented by each widget subclass.
Reimplemented from NvUIElement.
virtual float NvUIProxy::GetAlpha | ( | ) | [inline, virtual] |
virtual float NvUIProxy::GetHeight | ( | ) | [inline, virtual] |
virtual void NvUIProxy::GetScreenRect | ( | NvUIRect & | rect | ) | [inline, virtual] |
Accessor to retrieve the UI-space NvUIRect for this element into a passed-in reference.
Reimplemented from NvUIElement.
virtual uint32_t NvUIProxy::GetSlideFocusGroup | ( | ) | [inline, virtual] |
uint32_t NvUIProxy::GetUID | ( | ) | [inline] |
While we DO have a UID for ourselves, we always return the UID of the proxy instead.
Reimplemented from NvUIElement.
virtual bool NvUIProxy::GetVisibility | ( | ) | [inline, virtual] |
virtual float NvUIProxy::GetWidth | ( | ) | [inline, virtual] |
virtual NvUIEventResponse NvUIProxy::HandleEvent | ( | const NvGestureEvent & | ev, | |
NvUST | timeUST, | |||
NvUIElement * | hasFocus | |||
) | [inline, virtual] |
Virtual user interaction handling method.
We implement a base version to return not-handled, so that non-interactive classes don't have to.
- Parameters:
-
ev The current NvGestureEvent to handle timeUST A timestamp for the current interaction [in,out] hasFocus The element that had interaction last, or has taken over current interaction.
Reimplemented from NvUIElement.
Reimplemented in NvTweakVarUIProxyBase, and NvTweakEnumUI< T >.
virtual NvUIEventResponse NvUIProxy::HandleReaction | ( | const NvUIReaction & | react | ) | [inline, virtual] |
Virtual method for handling NvUIReaction in response to user interaction.
We implement a base version to return not-handled, so that non-reacting classes don't have to.
Reimplemented from NvUIElement.
Reimplemented in NvTweakVarUIProxyBase, NvTweakVarUI< T >, NvTweakEnumUI< T >, and NvTweakVarUI< T >.
virtual bool NvUIProxy::HasDepth | ( | ) | [inline, virtual] |
Method to test whether the element's bounding rect has a non-zero Z/depth value.
Reimplemented from NvUIElement.
virtual bool NvUIProxy::Hit | ( | float | x, | |
float | y | |||
) | [inline, virtual] |
Hit-test a given point against this element's UI rectangle, using no extra margin.
Reimplemented from NvUIElement.
virtual void NvUIProxy::LostFocus | ( | ) | [inline, virtual] |
Virtual method for telling this element it has lost interaction-focus.
Reimplemented from NvUIElement.
virtual void NvUIProxy::SetAlpha | ( | float | a | ) | [inline, virtual] |
virtual void NvUIProxy::SetDepth | ( | float | z | ) | [inline, virtual] |
virtual void NvUIProxy::SetDimensions | ( | float | w, | |
float | h | |||
) | [inline, virtual] |
Virtual method for setting the dimensions of this element in pixels.
Base implementation simply sets the NvUIElements rectangle width and height to passed in values.
Reimplemented from NvUIElement.
virtual void NvUIProxy::SetDrawState | ( | uint32_t | n | ) | [inline, virtual] |
Set the current drawing 'state' or index.
Primarily used for multi-state objects like Buttons to have active vs selected/highlighted, vs inactive states tracked, and those states can then be used to render different visuals.
Reimplemented from NvUIElement.
virtual void NvUIProxy::SetOrigin | ( | float | x, | |
float | y | |||
) | [inline, virtual] |
Virtual method for setting 2D origin point in UI/view space.
Base implementation simply sets the NvUIElement's rectangle top-left to the passed in values. Virtual as some subclasses may override to reposition children or account for padding/margins.
Reimplemented from NvUIElement.
virtual void NvUIProxy::SetVisibility | ( | bool | show | ) | [inline, virtual] |
The documentation for this class was generated from the following file: