NvTweakVar< T > Struct Template Reference
Templated class for holding a reference to a variable of a particular datatype. More...
#include <NvTweakVar.h>
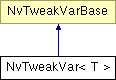
Public Member Functions | |
operator T & () | |
Value-reference operators to access the internal variable we manage. | |
operator const T & () const | |
Const value-reference operators to access the internal variable we manage. | |
const NvTweakVar & | operator= (T val) |
Assignment operator to set (once) the internal variable we will point to. | |
NvTweakVar (T &refVal, const char *name, T minVal, T maxVal, T step, char *description=NULL) | |
Clamped constructor, typically used for scalar variables. | |
NvTweakVar (const char *name, T minVal, T maxVal, T step, char *description=NULL) | |
Clamped constructor, used for self-referential scalars. | |
NvTweakVar (T &refVal, const char *name, const char *description=NULL) | |
Specialized unclamped constructor, generally used for bool variable. | |
NvTweakVar (const char *name, const char *description=NULL) | |
Specialized unclamped constructor, used for self-referential bool. | |
void | setValLoop (bool loop) |
Set whether or not to loop clamped value when increment/decrement reach ends of range. | |
virtual void | increment () |
Specific implementation of increment for the templated datatype. | |
virtual void | decrement () |
Specific implementation of decrement for the templated datatype. | |
virtual void | reset () |
Reset the managed variable to its initial value. | |
Protected Attributes | |
T & | mValRef |
Reference to variable being tracked/tweaked. | |
T | mValInitial |
Initial value, useful for 'reset' to starting point. | |
T | mValSelf |
A member of our datatype, for self-referencing NvTweakVar to point into itself. | |
T | mValMin |
Minimum value for a variable with clamped range. | |
T | mValMax |
Maximum value for a variable with clamped range. | |
T | mValStep |
Value step for the variable (increment/decrement). | |
bool | mValClamped |
Whether value adjustments to this variable are clamped/bounded. | |
bool | mValLoop |
When value is clamped, does value loop around when increment/decrement hits 'ends'. |
Detailed Description
template<class T>
struct NvTweakVar< T >
Templated class for holding a reference to a variable of a particular datatype.
Constructor & Destructor Documentation
NvTweakVar< T >::NvTweakVar | ( | T & | refVal, | |
const char * | name, | |||
T | minVal, | |||
T | maxVal, | |||
T | step, | |||
char * | description = NULL | |||
) | [inline] |
Clamped constructor, typically used for scalar variables.
NvTweakVar< T >::NvTweakVar | ( | const char * | name, | |
T | minVal, | |||
T | maxVal, | |||
T | step, | |||
char * | description = NULL | |||
) | [inline] |
Clamped constructor, used for self-referential scalars.
NvTweakVar< T >::NvTweakVar | ( | T & | refVal, | |
const char * | name, | |||
const char * | description = NULL | |||
) | [inline] |
Specialized unclamped constructor, generally used for bool variable.
NvTweakVar< T >::NvTweakVar | ( | const char * | name, | |
const char * | description = NULL | |||
) | [inline] |
Specialized unclamped constructor, used for self-referential bool.
Member Function Documentation
virtual void NvTweakVar< T >::decrement | ( | ) | [virtual] |
virtual void NvTweakVar< T >::increment | ( | ) | [virtual] |
NvTweakVar< T >::operator const T & | ( | ) | const [inline] |
Const value-reference operators to access the internal variable we manage.
NvTweakVar< T >::operator T & | ( | ) | [inline] |
Value-reference operators to access the internal variable we manage.
const NvTweakVar& NvTweakVar< T >::operator= | ( | T | val | ) | [inline] |
Assignment operator to set (once) the internal variable we will point to.
virtual void NvTweakVar< T >::reset | ( | ) | [inline, virtual] |
void NvTweakVar< T >::setValLoop | ( | bool | loop | ) | [inline] |
Set whether or not to loop clamped value when increment/decrement reach ends of range.
Member Data Documentation
bool NvTweakVar< T >::mValClamped [protected] |
Whether value adjustments to this variable are clamped/bounded.
T NvTweakVar< T >::mValInitial [protected] |
Initial value, useful for 'reset' to starting point.
bool NvTweakVar< T >::mValLoop [protected] |
When value is clamped, does value loop around when increment/decrement hits 'ends'.
T NvTweakVar< T >::mValMax [protected] |
Maximum value for a variable with clamped range.
T NvTweakVar< T >::mValMin [protected] |
Minimum value for a variable with clamped range.
T& NvTweakVar< T >::mValRef [protected] |
Reference to variable being tracked/tweaked.
T NvTweakVar< T >::mValSelf [protected] |
A member of our datatype, for self-referencing NvTweakVar to point into itself.
T NvTweakVar< T >::mValStep [protected] |
Value step for the variable (increment/decrement).
The documentation for this struct was generated from the following file: