nvImageCodec with cuPy#
[1]:
import os
import cv2
import cupy as cp
from matplotlib import pyplot as plt
Setting resource folder
[2]:
resources_dir = os.getenv("PYNVIMGCODEC_EXAMPLES_RESOURCES_DIR", "../assets/images/")
Import nvImageCodec module and create both Decoder and Encoder
[3]:
from nvidia import nvimgcodec
decoder = nvimgcodec.Decoder()
encoder = nvimgcodec.Encoder()
Load jpeg2000 image with nvImageCodec
[4]:
nv_img = decoder.read(resources_dir + "cat-1046544_640.jp2")
print(nv_img.__cuda_array_interface__)
{'shape': (475, 640, 3), 'strides': None, 'typestr': '|u1', 'data': (12918456320, False), 'version': 3, 'stream': 1}
Pass nvImageCodec Image to cupy asarray as it accepts __cuda_array_interface__
[5]:
%%time
cp_img = cp.asarray(nv_img)
print(cp_img.__cuda_array_interface__)
{'shape': (475, 640, 3), 'typestr': '|u1', 'descr': [('', '|u1')], 'stream': 1, 'version': 3, 'strides': None, 'data': (12918456320, False)}
CPU times: user 874 µs, sys: 638 µs, total: 1.51 ms
Wall time: 1.39 ms
Convert to numpy and show
[6]:
np_img4k = cp.asnumpy(cp_img)
plt.imshow(np_img4k)
[6]:
<matplotlib.image.AxesImage at 0x7f2d347c32e0>
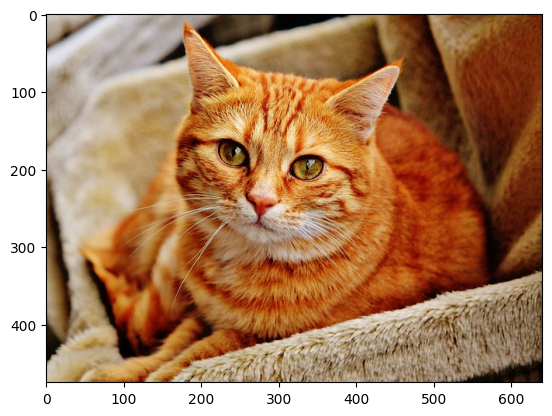
Lets do some opration on image in GPU using cupyx.scipy
[7]:
import cupyx.scipy.ndimage
[8]:
cp_img_rotated = cupyx.scipy.ndimage.rotate(cp_img, 90)
cp_img_gaussian = cupyx.scipy.ndimage.gaussian_filter(cp_img, sigma = 5)
print(cp_img_rotated.__cuda_array_interface__)
{'shape': (640, 475, 3), 'typestr': '|u1', 'descr': [('', '|u1')], 'stream': 1, 'version': 3, 'strides': None, 'data': (139827490676736, False)}
[9]:
np_img = cp.asnumpy(cp_img_rotated)
plt.imshow(np_img)
[9]:
<matplotlib.image.AxesImage at 0x7f2d317c05b0>
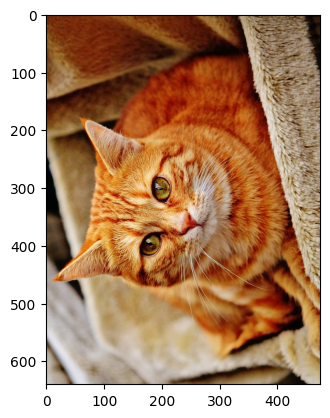
[10]:
np_img = cp.asnumpy(cp_img_gaussian)
plt.imshow(np_img)
[10]:
<matplotlib.image.AxesImage at 0x7f2c79d6d610>
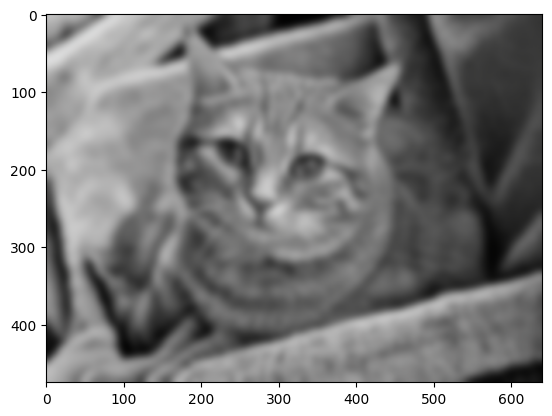
Convert cupy Image to nvImageCodec Image using __cuda_array_interface__
[11]:
%%time
nv_rotated_img = nvimgcodec.as_image(cp_img_rotated)
CPU times: user 46 µs, sys: 24 µs, total: 70 µs
Wall time: 73.4 µs
Check content
[12]:
plt.imshow(nv_rotated_img.cpu())
[12]:
<matplotlib.image.AxesImage at 0x7f2c79d62e20>
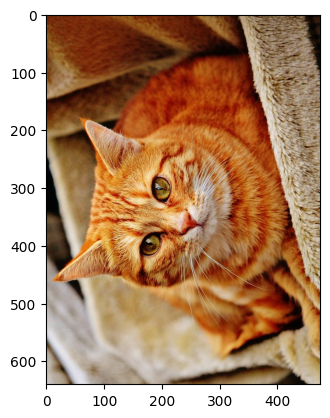
Save as Jpeg2000
[13]:
encoder.write("rotated.j2k", nv_rotated_img)
[13]:
'rotated.j2k'
Load with OpenCv to verify
[14]:
image = cv2.imread("rotated.j2k")
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
plt.imshow(image)
[14]:
<matplotlib.image.AxesImage at 0x7f2c79cd6cd0>
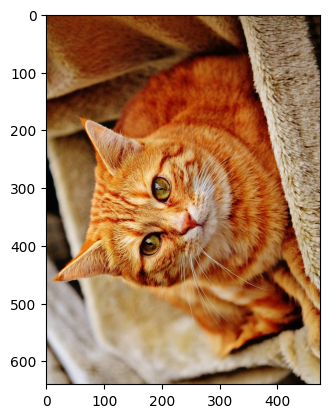
Save cupy image to jpg with nvImageCodec
[15]:
nv_img_gaussian = nvimgcodec.as_image(cp_img_gaussian)
encoder.write("gaussian.jpg", nv_img_gaussian)
[15]:
'gaussian.jpg'
Read back with OpenCV to verify
[16]:
image = cv2.imread("gaussian.jpg")
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
plt.imshow(image)
[16]:
<matplotlib.image.AxesImage at 0x7f2c79c54670>
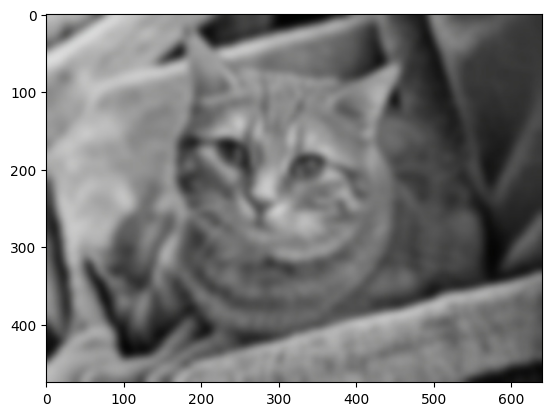
DLPack#
Passing cuPy ndarray to nvImageCodec using DLPack. cuPy ndarray has both cuda_array_interface and dlpack interface. By default, cuda_array_interface is preferred and will be used when we pass cuPy ndarray to nvImageCodec using as_image function. If we would like to use DLPack, firstly PyCapsule need to be taken from cuPy ndarray and then it needs to be passed to nvImageCodec.
[17]:
dlpack_img = cp_img_gaussian.toDlpack()
nv_img_gaussian = nvimgcodec.as_image(dlpack_img)
encoder.write("gaussian_dlpack.jpg", nv_img_gaussian)
[17]:
'gaussian_dlpack.jpg'
[18]:
image = cv2.imread("gaussian_dlpack.jpg")
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
plt.imshow(image)
[18]:
<matplotlib.image.AxesImage at 0x7f2c79bc54c0>
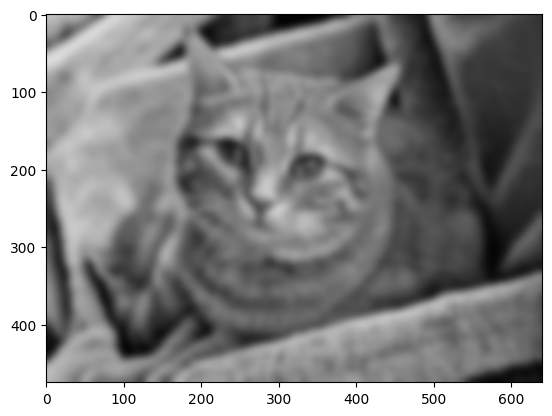
Passing nvImageCodec Image to cuPy using DLPack
[19]:
nv_img = decoder.read(resources_dir + "cat-1046544_640.jp2")
cp_img = cp.from_dlpack(nv_img)
[20]:
np_img = cp.asnumpy(cp_img)
plt.imshow(np_img)
[20]:
<matplotlib.image.AxesImage at 0x7f2c79b3d340>
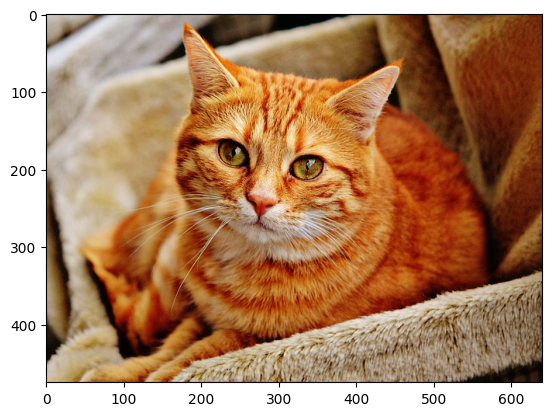
Alternatively, there is possibility to firstly take PyCapsule and then pass it to cuPy
[21]:
nv_img = decoder.read(resources_dir + "cat-1046544_640.jp2")
py_cap = nv_img.__dlpack__()
cp_img = cp.from_dlpack(py_cap)
[22]:
np_img = cp.asnumpy(cp_img)
plt.imshow(np_img4k)
[22]:
<matplotlib.image.AxesImage at 0x7f2c79ab3a60>
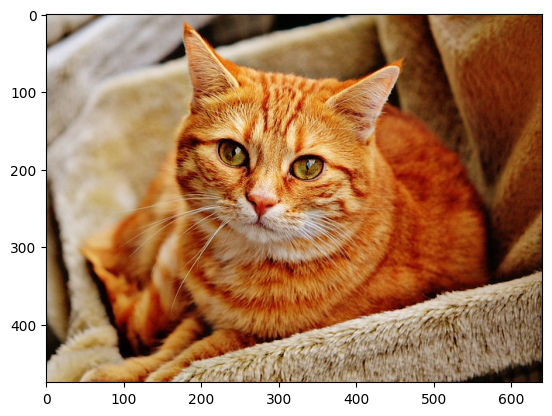