NvUIButton Class Reference
A class that implements a variety of standard interactive button widgets. More...
#include <NvUI.h>
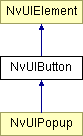
Public Member Functions | |
NvUIButton (NvUIButtonType::Enum btntype, uint32_t actionCode, NvUIElement *visrep[NvUIButtonState::MAX], const char *title=NULL, float pt=0, bool shadowed=false) | |
Most common constructor for a graphical, multi-state visual button. | |
NvUIButton (NvUIButtonType::Enum btntype, uint32_t actionCode, NvUIElement *visrep, const char *title=NULL, float pt=0, bool shadowed=false) | |
Button constructor for a quick, single-visual-state button. | |
NvUIButton (NvUIButtonType::Enum btntype, uint32_t actionCode, NvUIRect &size, const char *title, float pt, bool shadowed=false) | |
Button constructor for a quick, text-only button. | |
virtual | ~NvUIButton () |
Default destructor, cleans up visrep elements and title, if any. | |
virtual void | SetTitle (const char *title, float ptsize, bool shadowed=false) |
Set the title string of the button. | |
virtual void | SetTitleColor (const NvPackedColor &c) |
Set the color of the title text. | |
virtual void | SetHitMargin (float hitwide, float hittall) |
Add extra horizontal/vertical margin to thumb hit rect to make it easier to grab. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Draw the right UI element for current state, as well as optional title text. | |
virtual void | SetOrigin (float x, float y) |
Position sub-elements and optional title based on the button type. | |
virtual NvUIEventResponse | HandleEvent (const NvGestureEvent &ev, NvUST timeUST, NvUIElement *hasFocus) |
Handles tracking from press to release on the button object, and if needed posts appropriate NvUIReaction. | |
virtual NvUIEventResponse | HandleReaction (const NvUIReaction &react) |
Handles any reaction matching our action code. | |
virtual void | LostFocus () |
We override to clear hit tracking state, and reset draw state for push buttons. | |
uint32_t | GetActionCode () |
Accessor to get the reaction action code for this button. | |
void | SetSubCode (uint32_t c) |
Accessor to set the reaction sub-code for this button. | |
uint32_t | GetSubCode () |
Accessor to get the reaction sub-code for this button. | |
void | SetStickyClick (bool b) |
Set whether to retain the pressed-in even if user drags outside the hit rect. | |
void | ConsumeGesture (const NvGestureEvent &ev) |
Allow us to flag that we reacted to this gesture, so we don't handle again until new gesture UID. | |
Protected Attributes | |
NvUIElement * | m_visrep [NvUIButtonState::MAX] |
Abstract UI element array of visual representations we proxy as our button visual. | |
NvUIText * | m_title |
UIText-drawn title for a button, if one isn't baked into the graphic rep. | |
NvUIButtonType::Enum | m_type |
The 'class' of button (which defines its 'style' of interaction): push, radio, or checkbox. | |
uint32_t | m_action |
The reaction code 'posted' by the event handler when the button is pressed and released such that state changed. | |
uint32_t | m_subcode |
A specialized reaction code used by some kinds of buttons (radio in particular) to denote which sub-item was picked by index. | |
NvGestureUID | m_reactGestureUID |
A gesture event UID of the last gesture that we triggered/reacted to, so we can quickly ignore further HandleEvent calls until the gesture UID increments. | |
NvGestureUID | m_failedHitUID |
A gesture event UID of the last gesture that failed to hit the button, so we can quickly ignore further HandleEvent calls until the gesture UID increments. | |
bool | m_wasHit |
Used by HandleEvent and internal hit-tracking. | |
float | m_hitMarginWide |
An extra 'padding' or margin added to the button's normal hit-test width, in order to allow for a larger test region than the button's visual. | |
float | m_hitMarginTall |
An extra 'padding' or margin added to the button's normal hit-test height, in order to allow for a larger test region than the button's visual. | |
bool | m_stickyClick |
Whether to retain the pressed-in state even if we drag outside bounds. |
Detailed Description
A class that implements a variety of standard interactive button widgets.This class uses abstract visual representations of the three button states, combined with the logic behind the types of interactions of the three types of buttons, and the concept in the system of a 'reaction' to interacting with a button, and wraps it all up with end-to-end event handling.
This can represent a single graphical or textual (or other) element on the screen that is clickable.
While tracking a press within the button, the HandleEvent
method will attempt to keep the focus on the button, so that it will get 'first shot' at further event input in the system.
- See also:
- NvUIElement
Constructor & Destructor Documentation
NvUIButton::NvUIButton | ( | NvUIButtonType::Enum | btntype, | |
uint32_t | actionCode, | |||
NvUIElement * | visrep[NvUIButtonState::MAX], | |||
const char * | title = NULL , |
|||
float | pt = 0 , |
|||
bool | shadowed = false | |||
) |
Most common constructor for a graphical, multi-state visual button.
This is the generally 'best' method for building a visual button, as you give it the specific visual elements built for each state (active, pressed, inactive), and thus have very precise control over the visual results of interaction. Usually the visuals are an NvUIGraphic or NvUIGraphicFrame, but they can be any UI element (including containers with complex overlaid visuals, or custom visual subclasses).
- Parameters:
-
btntype The type/style of button actionCode The action code we raise in an NvUIReaction when our state changes visrep Array of UI elements that are our visuals for each interaction state, could have text embedded. title [optional] String to display for our title pt [optional] Font size to use if we supply title string shadowed [optional] Whether to drop-shadow our title text
- See also:
- NvUIGraphic
NvUIButton::NvUIButton | ( | NvUIButtonType::Enum | btntype, | |
uint32_t | actionCode, | |||
NvUIElement * | visrep, | |||
const char * | title = NULL , |
|||
float | pt = 0 , |
|||
bool | shadowed = false | |||
) |
Button constructor for a quick, single-visual-state button.
This button will not show different visuals while interacting, but otherwise has the ability to display any graphics/text to display as a button.
- Parameters:
-
btntype The type/style of button actionCode The action code we raise in an NvUIReaction when our state changes visrep The UI element that is our visual representation, could have text embedded. title [optional] String to display for our title pt [optional] Font size to use if we supply title string shadowed [optional] Whether to drop-shadow our title text
NvUIButton::NvUIButton | ( | NvUIButtonType::Enum | btntype, | |
uint32_t | actionCode, | |||
NvUIRect & | size, | |||
const char * | title, | |||
float | pt, | |||
bool | shadowed = false | |||
) |
Button constructor for a quick, text-only button.
- Parameters:
-
btntype The type/style of button actionCode The action code we raise in an NvUIReaction when our state changes size The UI/view space rectangle to position the button title The string to display for our title pt The font size to use for our title shadowed [optional] Whether to drop-shadow our title text
virtual NvUIButton::~NvUIButton | ( | ) | [virtual] |
Default destructor, cleans up visrep elements and title, if any.
Member Function Documentation
void NvUIButton::ConsumeGesture | ( | const NvGestureEvent & | ev | ) | [inline] |
Allow us to flag that we reacted to this gesture, so we don't handle again until new gesture UID.
virtual void NvUIButton::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
Draw the right UI element for current state, as well as optional title text.
Reimplemented from NvUIElement.
Reimplemented in NvUIPopup.
uint32_t NvUIButton::GetActionCode | ( | ) | [inline] |
Accessor to get the reaction action code for this button.
uint32_t NvUIButton::GetSubCode | ( | ) | [inline] |
Accessor to get the reaction sub-code for this button.
virtual NvUIEventResponse NvUIButton::HandleEvent | ( | const NvGestureEvent & | ev, | |
NvUST | timeUST, | |||
NvUIElement * | hasFocus | |||
) | [virtual] |
Handles tracking from press to release on the button object, and if needed posts appropriate NvUIReaction.
Reimplemented from NvUIElement.
Reimplemented in NvUIPopup.
virtual NvUIEventResponse NvUIButton::HandleReaction | ( | const NvUIReaction & | react | ) | [virtual] |
Handles any reaction matching our action code.
If the action matches our code, but the uid is zero, we assume it's a 'system message' telling us to override our state to what's in the reaction. Otherwise, if we're a radio button and receive our code, but not our uid, we assume another radio button got pressed, and we clear our state back to active.
Reimplemented from NvUIElement.
Reimplemented in NvUIPopup.
virtual void NvUIButton::LostFocus | ( | ) | [virtual] |
We override to clear hit tracking state, and reset draw state for push buttons.
Reimplemented from NvUIElement.
virtual void NvUIButton::SetHitMargin | ( | float | hitwide, | |
float | hittall | |||
) | [virtual] |
Add extra horizontal/vertical margin to thumb hit rect to make it easier to grab.
virtual void NvUIButton::SetOrigin | ( | float | x, | |
float | y | |||
) | [virtual] |
Position sub-elements and optional title based on the button type.
Reimplemented from NvUIElement.
Reimplemented in NvUIPopup.
void NvUIButton::SetStickyClick | ( | bool | b | ) | [inline] |
Set whether to retain the pressed-in even if user drags outside the hit rect.
void NvUIButton::SetSubCode | ( | uint32_t | c | ) | [inline] |
Accessor to set the reaction sub-code for this button.
virtual void NvUIButton::SetTitle | ( | const char * | title, | |
float | ptsize, | |||
bool | shadowed = false | |||
) | [virtual] |
Set the title string of the button.
Is used by constructors to set up our text string, but can be used to re-set the title later on.
- Parameters:
-
title The string to display for our title ptsize The font size to use for our title. Note if point size is passed in as 0, a size will be selected based on the height of the element. shadowed [optional] Whether to drop-shadow our title text
virtual void NvUIButton::SetTitleColor | ( | const NvPackedColor & | c | ) | [virtual] |
Set the color of the title text.
Member Data Documentation
uint32_t NvUIButton::m_action [protected] |
The reaction code 'posted' by the event handler when the button is pressed and released such that state changed.
Radio buttons use this to both notify change up the tree AND to notify 'linked' alternate state buttons to turn themselves back 'off'.
NvGestureUID NvUIButton::m_failedHitUID [protected] |
A gesture event UID of the last gesture that failed to hit the button, so we can quickly ignore further HandleEvent
calls until the gesture UID increments.
float NvUIButton::m_hitMarginTall [protected] |
An extra 'padding' or margin added to the button's normal hit-test height, in order to allow for a larger test region than the button's visual.
Important for touchscreen+finger interactivity.
float NvUIButton::m_hitMarginWide [protected] |
An extra 'padding' or margin added to the button's normal hit-test width, in order to allow for a larger test region than the button's visual.
Important for touchscreen+finger interactivity.
NvGestureUID NvUIButton::m_reactGestureUID [protected] |
A gesture event UID of the last gesture that we triggered/reacted to, so we can quickly ignore further HandleEvent
calls until the gesture UID increments.
bool NvUIButton::m_stickyClick [protected] |
Whether to retain the pressed-in state even if we drag outside bounds.
uint32_t NvUIButton::m_subcode [protected] |
A specialized reaction code used by some kinds of buttons (radio in particular) to denote which sub-item was picked by index.
NvUIText* NvUIButton::m_title [protected] |
UIText-drawn title for a button, if one isn't baked into the graphic rep.
NvUIButtonType::Enum NvUIButton::m_type [protected] |
The 'class' of button (which defines its 'style' of interaction): push, radio, or checkbox.
NvUIElement* NvUIButton::m_visrep[NvUIButtonState::MAX] [protected] |
Abstract UI element array of visual representations we proxy as our button visual.
Must have at least the 'active' state defined. Can be text, graphics, complex container, etc.
bool NvUIButton::m_wasHit [protected] |
Used by HandleEvent
and internal hit-tracking.
Set when we had a successful press/click in the thumb, so we are then tracking for drag/flick/release.
The documentation for this class was generated from the following file: