NvTweakBar Class Reference
Class representing a visual 'bar' of widgets (a 'tweakbar'). More...
#include <NvTweakBar.h>
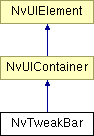
Public Member Functions | |
NvTweakBar (float width, float height) | |
Normal constructor. | |
virtual | ~NvTweakBar () |
Normal virtual destructor. | |
float | GetDefaultLineHeight () |
Accessor to retrieve the default height of one row in the tweakbar. | |
float | GetDefaultLineWidth () |
Accessor to retrieve the default width of a row in the tweakbar. | |
float | GetStartOffY () |
Accessor to retrieve the starting vertical offset of elements in the tweakbar. | |
virtual NvUIEventResponse | HandleReaction (const NvUIReaction &react) |
Method to handle results of user interaction with widgets. | |
virtual void | HandleReshape (float w, float h) |
Method to handle the effect of window/screen resize. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Method to handle drawing us on screen. | |
void | syncValues () |
Method to notify tweakbar widgets of possible changes to tracked variables. | |
void | setCompactLayout (bool compact) |
Method to request the tweakbar try to use more compact versions of widgets, and spacing between widgets, when possible. | |
void | textShadows (bool b) |
Method to request automatic dropshadows for all tweakbar text. | |
Methods for grouping together a block of related variables. | |
void | subgroupStart () |
Method to request the start of a set of widgets be grouped together. | |
void | subgroupEnd () |
Method to note the end of a grouped set of widgets. | |
void | subgroupSwitchStart (NvTweakVarBase *var) |
Method to request the start of a group of widgets, whose visibility is controlled by the value of a given NvTweakVarBase being tracked. | |
void | subgroupSwitchCase (bool val) |
Method for setting a case for a boolean tracked variable, with the value for the specific case. | |
void | subgroupSwitchCase (float val) |
Method for setting a case for a floating-point tracked variable, with the value for the specific case to match upon. | |
void | subgroupSwitchCase (uint32_t val) |
Method for setting a case for an integral tracked variable, with the value for the specific case to match upon. | |
void | subgroupSwitchEnd () |
Method to note the end of the current subgroup 'switch' block. | |
Methods for adding variables to the tweakbar. | |
void | addPadding () |
Requests vertical padding be added prior to the next widget or subgroup. | |
void | addLabel (const char *title, bool bold=false) |
Add a static text label to the tweakbar. | |
NvTweakVarBase * | addValue (const char *name, bool &var, bool pushButton=false, uint32_t actionCode=0) |
Add a bool variable to be controlled by the user. | |
NvTweakVarBase * | addButton (const char *name, uint32_t actionCode) |
Add an action-only push-button to the tweakbar. | |
NvTweakVarBase * | addValue (const char *name, float &var, float min, float max, float step=0, uint32_t actionCode=0) |
Add a floating-point variable to the tweakbar as a slider. | |
NvTweakVarBase * | addValue (const char *name, uint32_t &var, uint32_t min, uint32_t max, uint32_t step=0, uint32_t actionCode=0) |
Add an integral variable to the tweakbar as a slider. | |
NvTweakVarBase * | addEnum (const char *name, uint32_t &var, NvTweakEnum< uint32_t > values[], uint32_t valueCount, uint32_t actionCode=0) |
Add an integral variable to the tweakbar as an radio group or dropdown menu with an enumerated set of values. | |
NvTweakVarBase * | addMenu (const char *name, uint32_t &var, NvTweakEnum< uint32_t > values[], uint32_t valueCount, uint32_t actionCode=0) |
Add an integral variable to the tweakbar as a dropdown menu with an enumerated set of values. | |
Static Public Member Functions | |
static NvTweakBar * | CreateTweakBar (NvUIWindow *window) |
Static method to create an NvTweakBar attached to a window. |
Detailed Description
Class representing a visual 'bar' of widgets (a 'tweakbar').This class shows a contained group of user-interface widgets specific to the given application, for directly interacting with core variables controlling the application's functionality.
Constructor & Destructor Documentation
NvTweakBar::NvTweakBar | ( | float | width, | |
float | height | |||
) |
Normal constructor.
Note the constructor should not need to be manually called unless you are creating a very customized user interface which is not relying on NvSampleApp, nor CreateTweakBar to link up with an NvUIWindow and auto-size the tweakbar to fit reasonably.
- Parameters:
-
width The width of the bar in pixels height The height of the bar in pixels
virtual NvTweakBar::~NvTweakBar | ( | ) | [virtual] |
Normal virtual destructor.
Note the destructor should not need to be manually called unless you are creating a custom user interface not relying on NvSampleApp.
Member Function Documentation
NvTweakVarBase* NvTweakBar::addButton | ( | const char * | name, | |
uint32_t | actionCode | |||
) |
Add an action-only push-button to the tweakbar.
This method adds a push-button to the tweakbar that is not tied to an application variable. Instead, it simply generates the given action code for a normal NvUIReaction handling by the application -- and as such, the actionCode
parameter is required unlike other 'add' methods.
NvTweakVarBase* NvTweakBar::addEnum | ( | const char * | name, | |
uint32_t & | var, | |||
NvTweakEnum< uint32_t > | values[], | |||
uint32_t | valueCount, | |||
uint32_t | actionCode = 0 | |||
) |
Add an integral variable to the tweakbar as an radio group or dropdown menu with an enumerated set of values.
This method tracks and modifies the supplied variable using the passed in array of NvTweakEnum string-value pairs as individual items. It will create a radio-button group for two or three enum values, and a dropdown menu for four or more values.
void NvTweakBar::addLabel | ( | const char * | title, | |
bool | bold = false | |||
) |
Add a static text label to the tweakbar.
NvTweakVarBase* NvTweakBar::addMenu | ( | const char * | name, | |
uint32_t & | var, | |||
NvTweakEnum< uint32_t > | values[], | |||
uint32_t | valueCount, | |||
uint32_t | actionCode = 0 | |||
) |
Add an integral variable to the tweakbar as a dropdown menu with an enumerated set of values.
This method tracks and modifies the supplied variable using the passed in array of NvTweakEnum string-value pairs as the menu items in a dropdown menu.
void NvTweakBar::addPadding | ( | ) |
Requests vertical padding be added prior to the next widget or subgroup.
NvTweakVarBase* NvTweakBar::addValue | ( | const char * | name, | |
uint32_t & | var, | |||
uint32_t | min, | |||
uint32_t | max, | |||
uint32_t | step = 0 , |
|||
uint32_t | actionCode = 0 | |||
) |
Add an integral variable to the tweakbar as a slider.
This method adds a slider to the tweakbar, tracking the supplied floating-point variable.
NvTweakVarBase* NvTweakBar::addValue | ( | const char * | name, | |
float & | var, | |||
float | min, | |||
float | max, | |||
float | step = 0 , |
|||
uint32_t | actionCode = 0 | |||
) |
Add a floating-point variable to the tweakbar as a slider.
This method adds a slider to the tweakbar, tracking the supplied floating-point variable.
NvTweakVarBase* NvTweakBar::addValue | ( | const char * | name, | |
bool & | var, | |||
bool | pushButton = false , |
|||
uint32_t | actionCode = 0 | |||
) |
Add a bool variable to be controlled by the user.
This method adds the given bool variable to be tracked by the system and controlled by the user. By default, the variable is bound to a checkbox interface (so users can toggle between true and false), but passing true for the pushButton
parameter will bind it to a push-button instead, only asserting a true value (relying on the application to handle and then reset the variable to false).
static NvTweakBar* NvTweakBar::CreateTweakBar | ( | NvUIWindow * | window | ) | [static] |
Static method to create an NvTweakBar attached to a window.
This method helps you to creates an instance of NvTweakBar that is properly connected up with the provided NvUIWindow.
- Parameters:
-
window The NvUIWindow to attach to.
virtual void NvTweakBar::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
Method to handle drawing us on screen.
- Parameters:
-
drawState The NvUIDrawState that encapsulates needed state.
Reimplemented from NvUIContainer.
float NvTweakBar::GetDefaultLineHeight | ( | ) | [inline] |
Accessor to retrieve the default height of one row in the tweakbar.
float NvTweakBar::GetDefaultLineWidth | ( | ) | [inline] |
Accessor to retrieve the default width of a row in the tweakbar.
float NvTweakBar::GetStartOffY | ( | ) | [inline] |
Accessor to retrieve the starting vertical offset of elements in the tweakbar.
Primary use of this method is so external controls can be aligned with the first line of the tweakbar, for visual continuity.
virtual NvUIEventResponse NvTweakBar::HandleReaction | ( | const NvUIReaction & | react | ) | [virtual] |
Method to handle results of user interaction with widgets.
- Parameters:
-
react The reaction structure to process/handle.
Reimplemented from NvUIContainer.
virtual void NvTweakBar::HandleReshape | ( | float | w, | |
float | h | |||
) | [virtual] |
Method to handle the effect of window/screen resize.
- Parameters:
-
w The new view width. h The new view height.
Reimplemented from NvUIContainer.
void NvTweakBar::setCompactLayout | ( | bool | compact | ) | [inline] |
Method to request the tweakbar try to use more compact versions of widgets, and spacing between widgets, when possible.
- Parameters:
-
compact Pass in true to enable compact layout logic.
void NvTweakBar::subgroupSwitchCase | ( | uint32_t | val | ) |
Method for setting a case for an integral tracked variable, with the value for the specific case to match upon.
- Parameters:
-
val The integral case value for this subgroup/block.
void NvTweakBar::subgroupSwitchCase | ( | float | val | ) |
Method for setting a case for a floating-point tracked variable, with the value for the specific case to match upon.
- Parameters:
-
val The floating-point case value for this subgroup/block.
void NvTweakBar::subgroupSwitchCase | ( | bool | val | ) |
Method for setting a case for a boolean tracked variable, with the value for the specific case.
- Parameters:
-
val The boolean case value for this subgroup/block.
void NvTweakBar::subgroupSwitchStart | ( | NvTweakVarBase * | var | ) |
Method to request the start of a group of widgets, whose visibility is controlled by the value of a given NvTweakVarBase being tracked.
This method starts a very special grouping of widgets, working like a 'case' statement, where each case/subgroup is only visible when the tracked variable matches the value of the given subgroup's case.
An example use might be something like the following:
// A temporary variable to hold the current NvTweakVarBase we're referencing. NvTweakVarBase *var; // Add a variable to be managed by the tweakbar, and get the returned pointer var = mTweakBar->addValue("Auto LOD", mLod); // Start a 'switch' based on the returned variable mTweakBar->subgroupSwitchStart(var); // Handle the switch case for 'true' mTweakBar->subgroupSwitchCase(true); // Add a variable to the current switch case. var = mTweakBar->addValue("Triangle size", mParams.triSize, 1.0f, 50.0f); // Handle the switch case for 'false' mTweakBar->subgroupSwitchCase(false); // Add a variable to the current switch case. var = mTweakBar->addValue("Inner tessellation factor", mParams.innerTessFactor, 1.0f, 64.0f); // Add another variable to the current case. var = mTweakBar->addValue("Outer tessellation factor", mParams.outerTessFactor, 1.0f, 64.0f); // Close/end the switch group. mTweakBar->subgroupSwitchEnd();
- Parameters:
-
var The NvTweakVarBase object to track and switch visibility upon.
void NvTweakBar::syncValues | ( | ) |
Method to notify tweakbar widgets of possible changes to tracked variables.
This method is called to let the tweakbar know that some outside system has (potentially) changed the underlyign values of variables that tweakbar widgets are also watching/controlling via NvTweakVars, and thus all widgets should go and refresh themselves.
void NvTweakBar::textShadows | ( | bool | b | ) | [inline] |
Method to request automatic dropshadows for all tweakbar text.
- Parameters:
-
b Pass true to enable automatic dropshadows. Default is false.
The documentation for this class was generated from the following file: