NvUIContainer Class Reference
An object that holds and manages a group of UI elements and interactions with them. More...
#include <NvUI.h>
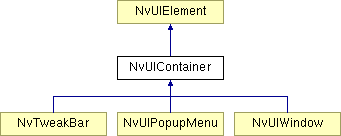
Public Member Functions | |
NvUIContainer (float width, float height, NvUIGraphic *bg=NULL) | |
Normal constructor. | |
virtual | ~NvUIContainer () |
Normal virtual destructor. | |
void | SetBackground (NvUIGraphic *bg) |
Set the background graphic element to draw before all children. | |
void | SetConsumeClicks (bool b) |
Set whether or not to consume all unhandled input within our bounding rect. | |
virtual void | Add (NvUIElement *el, float desiredleft, float desiredtop) |
Add a child element to our list, at the desired top/left offset. | |
virtual bool | Remove (NvUIElement *child) |
Remove a child from our list. | |
virtual void | AddPopup (NvUIElement *el) |
Hold onto a specific UI element to be drawn 'popped up' on top of all other elements. | |
virtual void | RemovePopup (NvUIElement *el) |
Clear prior popped-up element. | |
virtual void | SetOrigin (float x, float y) |
Set the top-left origin point of our bounding rect. | |
virtual void | SetDimensions (float w, float h) |
Set width/height dimensions of our bounding rect. | |
virtual NvUIEventResponse | HandleEvent (const NvGestureEvent &ev, NvUST timeUST, NvUIElement *hasFocus) |
Implements dispatching HandleEvent calls through to all contained children. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Draws a backgound if we have one, followed by children in order of the linked-list. | |
virtual NvUIEventResponse | HandleReaction (const NvUIReaction &react) |
Implements dispatching HandleReaction calls through to all contained children. | |
virtual void | HandleReshape (float w, float h) |
Handle change in view size. | |
virtual void | LostFocus () |
Clear any state related to interaction focus. | |
virtual bool | MakeChildFrontmost (NvUIElement *child) |
Move a specified child to be drawn in front of all others. | |
NvUIElement * | GetFocusedChild () |
Accessor for pointer to the child currently being interacted with. | |
virtual uint32_t | GetSlideFocusGroup () |
Accessor for the SlideFocusGroup identifier. | |
Protected Attributes | |
NvUIGraphic * | m_background |
A background visual to draw behind all children, stretched to fill our dimensions. | |
bool | m_consumeClicks |
A flag for whether to report events inside the container as handled even if no child wants them -- thus 'consuming' them and preventing others from trying to use them. | |
NvUIElement * | m_childrenHead |
Head of a doubly-linked, non-circular list of child elements. | |
NvUIElement * | m_childrenTail |
Tail of a doubly-linked list of children. | |
uint32_t | m_numChildren |
Number of child element on our linked-list. | |
NvUIElement * | m_focusedChild |
The child that the user has most recently interacted with. | |
NvUIElement * | m_popup |
A temporary (short-lived), popped-up element that we want drawn over everything else. |
Detailed Description
An object that holds and manages a group of UI elements and interactions with them.This class implements a 'container' or 'holder' object, that has no particular visual of its own, rather 'proxying' its visual to the children held within it. It does do custom handling for having a specific 'background' visual attached to it (such as a graphic or a frame) to draw beneath the children.
The main interface is the Add
method, which allows you to generally add any kind of UI element into the container, at a specific position in space. The container, if moved, will properly adjust the position of all contained child UI elements to keep proper relative positioning between them. This allows for a container to be 'shifted' around (jumped, or slowly slid, for buttonbars or or 'submenus' or the like), as well as alpha-faded (as alpha in the drawstate is passed through to child Draw
calls), and hidden/shown (visibility control).
The container also has quite detailed handling of NvGestureEvents and NvUIReactions, actually implementing the main 'translation' between the two systems (that is, NvGestureEvents come in that a child might handle and issue an NvUIReaction to, and the container code passes the reaction up. The NvUIWindow class is a specialized container that automatically calls down into its children after event handling to allow them a chance at processing any Reaction, without further explicit code by the developer.
The container also specially manages the focus state, special-case handling any incoming events, and passing them to the last-focused child for a 'first shot' at those events before passing them to all children in general.
Constructor & Destructor Documentation
NvUIContainer::NvUIContainer | ( | float | width, | |
float | height, | |||
NvUIGraphic * | bg = NULL | |||
) |
Normal constructor.
Takes width and height dimensions, optional background graphic, and optional flags. Origin is set separately.
virtual NvUIContainer::~NvUIContainer | ( | ) | [virtual] |
Normal virtual destructor.
Member Function Documentation
virtual void NvUIContainer::Add | ( | NvUIElement * | el, | |
float | desiredleft, | |||
float | desiredtop | |||
) | [virtual] |
Add a child element to our list, at the desired top/left offset.
virtual void NvUIContainer::AddPopup | ( | NvUIElement * | el | ) | [virtual] |
Hold onto a specific UI element to be drawn 'popped up' on top of all other elements.
virtual void NvUIContainer::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
Draws a backgound if we have one, followed by children in order of the linked-list.
Reimplemented from NvUIElement.
Reimplemented in NvTweakBar.
NvUIElement* NvUIContainer::GetFocusedChild | ( | ) | [inline] |
Accessor for pointer to the child currently being interacted with.
virtual uint32_t NvUIContainer::GetSlideFocusGroup | ( | ) | [inline, virtual] |
virtual NvUIEventResponse NvUIContainer::HandleEvent | ( | const NvGestureEvent & | ev, | |
NvUST | timeUST, | |||
NvUIElement * | hasFocus | |||
) | [virtual] |
Implements dispatching HandleEvent calls through to all contained children.
- The focused child always gets an early shot at the event, in order to shortcut all further processing, since it was last to interact with the user and highly likely to handle the next event.
- If there is a popup attached to us, and was not the focused child, it gets the next shot at the event, ahead of the normal loop over all children.
- Lastly, we give the list of children a shot at the event. Note that we walk the child list tail-to-head, in order to process clicks frontmost-first.
- After all child processing is complete, if the event still has not been handled but SetConsumesClicks(true) was called on us, if the event was inside us and the start of or continuation of a chain of events, we will consume the event by returning it as having been handled by us.
Reimplemented from NvUIElement.
Reimplemented in NvUIWindow, and NvUIPopupMenu.
virtual NvUIEventResponse NvUIContainer::HandleReaction | ( | const NvUIReaction & | react | ) | [virtual] |
Implements dispatching HandleReaction calls through to all contained children.
As any contained NvUIElement might respond to the raised NvUIReaction, we walk the linked-list in normal head-to-tail order, since it is no more likely that any one element will handle the reaction.
Reimplemented from NvUIElement.
Reimplemented in NvTweakBar.
virtual void NvUIContainer::HandleReshape | ( | float | w, | |
float | h | |||
) | [inline, virtual] |
virtual void NvUIContainer::LostFocus | ( | ) | [virtual] |
virtual bool NvUIContainer::MakeChildFrontmost | ( | NvUIElement * | child | ) | [virtual] |
Move a specified child to be drawn in front of all others.
virtual bool NvUIContainer::Remove | ( | NvUIElement * | child | ) | [virtual] |
Remove a child from our list.
virtual void NvUIContainer::RemovePopup | ( | NvUIElement * | el | ) | [virtual] |
Clear prior popped-up element.
void NvUIContainer::SetBackground | ( | NvUIGraphic * | bg | ) | [inline] |
Set the background graphic element to draw before all children.
void NvUIContainer::SetConsumeClicks | ( | bool | b | ) | [inline] |
Set whether or not to consume all unhandled input within our bounding rect.
virtual void NvUIContainer::SetDimensions | ( | float | w, | |
float | h | |||
) | [virtual] |
Set width/height dimensions of our bounding rect.
These values are especially applicable if the container is flagged to clip children to its bounds.
Reimplemented from NvUIElement.
virtual void NvUIContainer::SetOrigin | ( | float | x, | |
float | y | |||
) | [virtual] |
Member Data Documentation
NvUIGraphic* NvUIContainer::m_background [protected] |
A background visual to draw behind all children, stretched to fill our dimensions.
NvUIElement* NvUIContainer::m_childrenHead [protected] |
Head of a doubly-linked, non-circular list of child elements.
The use of internal linked-list fields of NvUIElement means child can only exist in one container at any one time.
We walk the list forward to draw back-to-front visuals.
NvUIElement* NvUIContainer::m_childrenTail [protected] |
Tail of a doubly-linked list of children.
We need double-link so we can walk the list tail-to-head in order to process pointer events topmost-first.
bool NvUIContainer::m_consumeClicks [protected] |
A flag for whether to report events inside the container as handled even if no child wants them -- thus 'consuming' them and preventing others from trying to use them.
NvUIElement* NvUIContainer::m_focusedChild [protected] |
The child that the user has most recently interacted with.
Cleared if the child no longer responds that it wants focus, or if the container's LostFocus
method is explicitly called.
uint32_t NvUIContainer::m_numChildren [protected] |
Number of child element on our linked-list.
NvUIElement* NvUIContainer::m_popup [protected] |
A temporary (short-lived), popped-up element that we want drawn over everything else.
The documentation for this class was generated from the following file: