NvSampleApp Class Reference
Base class for sample apps. More...
#include <NvSampleApp.h>
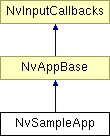
Public Member Functions | |
NvSampleApp (NvPlatformContext *platform, const char *appTitle=NULL) | |
Constructor Do NOT make rendering API calls in the constructor The rendering context is not bound until the entry into initRendering. | |
virtual | ~NvSampleApp () |
Destructor. | |
virtual void | initUI (void) |
UI init callback. | |
virtual void | drawUI (void) |
App-specific UI drawing callback. | |
virtual void | mainLoop () |
The base class provides an implementation of the mainloop that calls the virtual "callbacks" . | |
NvUIWindow * | getUIWindow () |
Get UI window. | |
NvFramerateCounter * | getFramerate () |
Get the framerate counter. | |
bool | requireExtension (const char *ext, bool exitOnFailure=true) |
Extension requirement declaration. | |
bool | requireMinAPIVersion (const NvGfxAPIVersion &minApi, bool exitOnFailure=true) |
GL Minimum API requirement declaration. | |
void | errorExit (const char *errorString) |
Exit with indication of an error Exits in all cases; in normal mode it shows the string in a dialog and exits In test mode, it writes the string to the log file and exits. | |
float | getFrameDeltaTime () |
Frame delta time. | |
void | addTweakKeyBind (NvTweakVarBase *var, uint32_t incKey, uint32_t decKey=0) |
Key binding. | |
void | addTweakButtonBind (NvTweakVarBase *var, uint32_t incBtn, uint32_t decBtn=0) |
Gamepad Button binding. | |
virtual bool | getRequestedWindowSize (int32_t &width, int32_t &height) |
Window size request. | |
virtual NvUIEventResponse | handleReaction (const NvUIReaction &react) |
Protected Types | |
typedef std::map< uint32_t, NvTweakBind > | NvAppKeyBind |
typedef std::map< uint32_t, NvTweakBind > | NvAppButtonBind |
Protected Member Functions | |
bool | isTestMode () |
Test mode query. | |
bool | pointerInput (NvInputDeviceType::Enum device, NvPointerActionType::Enum action, uint32_t modifiers, int32_t count, NvPointerEvent *points) |
Pointer input event. | |
bool | keyInput (uint32_t code, NvKeyActionType::Enum action) |
Key input event Called when a key is pressed, released or held param[in] code the keycode of the event. | |
bool | characterInput (uint8_t c) |
Character input event Called when a keypressed, release or hold is unhandled and maps to a character param[in] c the ASCII character of the event. | |
bool | gamepadChanged (uint32_t changedPadFlags) |
Gamepad input event Called when any button or axis value on any active gamepad changes. | |
bool | gamepadButtonChanged (uint32_t button, bool down) |
Protected Attributes | |
NvFramerateCounter * | mFramerate |
float | mFrameDelta |
NvStopWatch * | mFrameTimer |
NvUIWindow * | mUIWindow |
NvUIText * | mFPSText |
NvTweakBar * | mTweakBar |
NvUIButton * | mTweakTab |
NvInputTransformer * | m_transformer |
NvAppKeyBind | mKeyBinds |
NvAppButtonBind | mButtonBinds |
NvGamepad::State | mLastPadState [NvGamepad::MAX_GAMEPADS] |
Detailed Description
Base class for sample apps.Adds numerous features to NvAppBase that are of use to most or all sample apps
Constructor & Destructor Documentation
NvSampleApp::NvSampleApp | ( | NvPlatformContext * | platform, | |
const char * | appTitle = NULL | |||
) |
Constructor Do NOT make rendering API calls in the constructor The rendering context is not bound until the entry into initRendering.
- Parameters:
-
[in] platform the platform context representing the system, normally passed in from the NvAppFactory [in] appTitle the null-terminated string title of the application
Member Function Documentation
void NvSampleApp::addTweakButtonBind | ( | NvTweakVarBase * | var, | |
uint32_t | incBtn, | |||
uint32_t | decBtn = 0 | |||
) |
Gamepad Button binding.
Adds a button binding.
- Parameters:
-
[in] var the tweak variable to be bound [in] incKey the button to be bound to increment the tweak variable [in] decKey the button to be bound to decrement the tweak variable
void NvSampleApp::addTweakKeyBind | ( | NvTweakVarBase * | var, | |
uint32_t | incKey, | |||
uint32_t | decKey = 0 | |||
) |
Key binding.
Adds a key binding.
- Parameters:
-
[in] var the tweak variable to be bound [in] incKey the key to be bound to increment the tweak variable [in] decKey the key to be bound to decrement the tweak variable
bool NvSampleApp::characterInput | ( | uint8_t | c | ) | [protected, virtual] |
Character input event Called when a keypressed, release or hold is unhandled and maps to a character param[in] c the ASCII character of the event.
- Returns:
- true if the recipient handled the event, false if the recipient wishes the caller to handle the event
Implements NvInputCallbacks.
virtual void NvSampleApp::drawUI | ( | void | ) | [inline, virtual] |
App-specific UI drawing callback.
Called to request the app render any UI elements over the frame.
void NvSampleApp::errorExit | ( | const char * | errorString | ) |
Exit with indication of an error Exits in all cases; in normal mode it shows the string in a dialog and exits In test mode, it writes the string to the log file and exits.
- Parameters:
-
[in] errorString a null-terminated string indicating the error
bool NvSampleApp::gamepadChanged | ( | uint32_t | changedPadFlags | ) | [protected, virtual] |
Gamepad input event Called when any button or axis value on any active gamepad changes.
- Parameters:
-
[in] changedPadFlags a mask of the changed pad indices. For each gamepad i that has changed, bit (1<<i) will be set.
- Returns:
- true if the recipient handled the event, false if the recipient wishes the caller to handle the event
Implements NvInputCallbacks.
float NvSampleApp::getFrameDeltaTime | ( | ) | [inline] |
Frame delta time.
- Returns:
- the time since the last frame in seconds
NvFramerateCounter* NvSampleApp::getFramerate | ( | ) | [inline] |
Get the framerate counter.
The NvSampleApp initializes and updates an NvFramerateCounter in its mainloop implementation. It also draws it to the screen. The application may gain access if it wishes to get the data for its own use.
- Returns:
- a pointer to the framerate counter object
virtual bool NvSampleApp::getRequestedWindowSize | ( | int32_t & | width, | |
int32_t & | height | |||
) | [virtual] |
Window size request.
Allows the app to change the default window size. While an app can override this, it is NOT recommended, as the base class parses the command line arguments to set the window size. Applications wishing to override this should call the base class version and return without changing the values if the base class returns true. Application must return true if it changes the width or height passed in Not all platforms can support setting the window size. These platforms will not call this function Most apps should be resolution-agnostic and be able to run at a given resolution
- Parameters:
-
[in,out] width the default width is passed in. If the application wishes to reuqest it be changed, it should change the value before returning true [in,out] height the default height is passed in. If the application wishes to reuqest it be changed, it should change the value before returning true
- Returns:
- whether the value has been changed. true if changed, false if not
Reimplemented from NvAppBase.
NvUIWindow* NvSampleApp::getUIWindow | ( | ) | [inline] |
Get UI window.
- Returns:
- a pointer to the UI window
virtual void NvSampleApp::initUI | ( | void | ) | [inline, virtual] |
UI init callback.
Called after rendering is initialized, to allow preparation of overlaid UI elements
bool NvSampleApp::isTestMode | ( | ) | [inline, protected] |
Test mode query.
- Returns:
- true if the app is running in a timed test harness
bool NvSampleApp::keyInput | ( | uint32_t | code, | |
NvKeyActionType::Enum | action | |||
) | [protected, virtual] |
Key input event Called when a key is pressed, released or held param[in] code the keycode of the event.
This is an NvKey mask param[in] action the action for the given key
- Returns:
- true if the recipient handled the event, false if the recipient wishes the caller to handle the event. Returning true from a key event will generally preclude any characterInput events coming from the action
Implements NvInputCallbacks.
virtual void NvSampleApp::mainLoop | ( | ) | [virtual] |
The base class provides an implementation of the mainloop that calls the virtual "callbacks" .
Leaving this function as implemented in the App base class allows the application to simply override the individual callbacks to implement their app behavior. However, apps can still copy the source of the App::mainLoop into their own override of the function and either modify it slightly or completely replace it.
Reimplemented from NvAppBase.
bool NvSampleApp::pointerInput | ( | NvInputDeviceType::Enum | device, | |
NvPointerActionType::Enum | action, | |||
uint32_t | modifiers, | |||
int32_t | count, | |||
NvPointerEvent * | points | |||
) | [protected, virtual] |
Pointer input event.
Called when any pointer device has changed
- Parameters:
-
[in] device the device generating the event [in] action the action represented by the event [in] modifiers any modifiers to the event (normally only for mice) [in] count the number of points in the points array parameter [in] points an array of the points in the event (normally a single point unless the device is a multi-touch screen
- Returns:
- true if the recipient handled the event, false if the recipient wishes the caller to handle the event
Implements NvInputCallbacks.
bool NvSampleApp::requireExtension | ( | const char * | ext, | |
bool | exitOnFailure = true | |||
) |
Extension requirement declaration.
Allow an app to declare an extension as "required".
- Parameters:
-
[in] ext the extension name to be required [in] exitOnFailure if true, then errorExit is called to indicate the issue and exit
- Returns:
- true if the extension string is exported and false if it is not
bool NvSampleApp::requireMinAPIVersion | ( | const NvGfxAPIVersion & | minApi, | |
bool | exitOnFailure = true | |||
) |
GL Minimum API requirement declaration.
- Parameters:
-
[in] minApi the minimum API that is required [in] exitOnFailure if true, then errorExit is called to indicate the issue and exit
- Returns:
- true if the platform's GL[ES] API version is at least as new as the given minApi. Otherwise, returns false
The documentation for this class was generated from the following file: