NvAppBase Class Reference
A basic app framework, including mainloop, setup and input processing. More...
#include <NvAppBase.h>
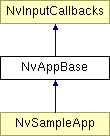
Public Member Functions | |
NvAppBase (NvPlatformContext *platform, const char *appTitle) | |
Constructor Do NOT make rendering API calls in the constructor The rendering context is not bound until the entry into initRendering. | |
virtual | ~NvAppBase () |
Destructor. | |
virtual void | configurationCallback (NvEGLConfiguration &config) |
GL configuration request callback. | |
NvGLAppContext * | getGLContext () |
Get GL context info. | |
void | setGLContext (NvGLAppContext *context) |
Set GL context info. | |
NvPlatformContext * | getPlatformContext () |
Get platform context. | |
bool | isMobilePlatform () |
Mobile platform query. | |
NvStopWatch * | createStopWatch () |
Create a StopWatch. | |
uint64_t | queryPerformanceCounter () |
Get high-performance timer value. | |
uint64_t | queryPerformanceCounterFrequency () |
Get high-performance timer frequency. | |
bool | showDialog (const char *title, const char *contents, bool exitApp) |
Show info/error dialog. | |
bool | writeScreenShot (int32_t width, int32_t height, const uint8_t *data, const std::string &path) |
Write RGB image to file Debugging function to write a block of RGB 24-bit data to an image file. | |
bool | writeLogFile (const std::string &path, bool append, const char *fmt,...) |
Write text to logging file. | |
void | forceLinkHack () |
Linker hack. | |
virtual void | mainLoop () |
Mainloop function. | |
virtual bool | getRequestedWindowSize (int32_t &width, int32_t &height) |
Window size request callback. | |
Mainloop Application Callbacks | |
To be overridden in concrete application subclasses. | |
virtual void | initRendering (void) |
Initialize rendering. | |
virtual void | shutdownRendering (void) |
Shutdown rendering. | |
virtual void | update (void) |
Application animation update. | |
virtual void | draw (void) |
Rendering callback. | |
virtual void | reshape (int32_t width, int32_t height) |
Resize callback. | |
virtual void | focusChanged (bool focused) |
Focus loss/gain callback. | |
InputCallback null implementations. | |
virtual bool | handlePointerInput (NvInputDeviceType::Enum device, NvPointerActionType::Enum action, uint32_t modifiers, int32_t count, NvPointerEvent *points) |
virtual bool | handleKeyInput (uint32_t code, NvKeyActionType::Enum action) |
virtual bool | handleCharacterInput (uint8_t c) |
virtual bool | handleGamepadChanged (uint32_t changedPadFlags) |
virtual bool | handleGamepadButtonChanged (uint32_t button, bool down) |
Protected Member Functions | |
void | appRequestExit () |
Request exit. | |
bool | isExiting () |
Exit query. | |
Protected Attributes | |
int32_t | m_width |
the current window width | |
int32_t | m_height |
the current window height |
Detailed Description
A basic app framework, including mainloop, setup and input processing.Constructor & Destructor Documentation
NvAppBase::NvAppBase | ( | NvPlatformContext * | platform, | |
const char * | appTitle | |||
) |
Constructor Do NOT make rendering API calls in the constructor The rendering context is not bound until the entry into initRendering.
- Parameters:
-
[in] platform the platform context representing the system, normally passed in from the NvAppFactory [in] appTitle the null-terminated string title of the application
Member Function Documentation
void NvAppBase::appRequestExit | ( | ) | [protected] |
Request exit.
Called by the application subclass if it wishes to exit/quit
virtual void NvAppBase::configurationCallback | ( | NvEGLConfiguration & | config | ) | [inline, virtual] |
GL configuration request callback.
This function passes in the default set of GL configuration requests The app can override this function and change the defaults before returning. These are still requests, and may not be met. If the platform supports requesting GL options, this function will be called before initGL. Optional.
- Parameters:
-
[in,out] config the default config to be used is passed in. If the application wishes anything different in the GL configuration, it should change those values before returning from the function. These are merely requests.
NvStopWatch* NvAppBase::createStopWatch | ( | ) |
Create a StopWatch.
- Returns:
- a pointer to a valid stopwatch object or NULL on failure
virtual void NvAppBase::draw | ( | void | ) | [inline, virtual] |
Rendering callback.
Called to request the app render a frame at regular intervals when the app is focused or when force by outside events like a resize
virtual void NvAppBase::focusChanged | ( | bool | focused | ) | [inline, virtual] |
Focus loss/gain callback.
Called to indicate that the app's window has gained/lost focus
- Parameters:
-
[in] focused true if application is gaining focus, false if it is losing focus
void NvAppBase::forceLinkHack | ( | ) |
Linker hack.
An empty function that ensures the linker does not strip the framework
NvGLAppContext* NvAppBase::getGLContext | ( | ) | [inline] |
Get GL context info.
- Returns:
- the GL context info. This will be NULL at app startup, and is likely to be NULL on some platforms until shouldRender returns true
NvPlatformContext* NvAppBase::getPlatformContext | ( | ) | [inline] |
Get platform context.
- Returns:
- the platform context object. This will be valid for the life of the App
virtual bool NvAppBase::getRequestedWindowSize | ( | int32_t & | width, | |
int32_t & | height | |||
) | [inline, virtual] |
Window size request callback.
Application must return true if it changes the width or height passed in Not all platforms can support setting the window size. These platforms will not call this function Most apps should be resolution-agnostic and be able to run at a given resolution
- Parameters:
-
[in,out] width the default width is passed in. If the application wishes to reuqest it be changed, it should change the value before returning true [in,out] height the default height is passed in. If the application wishes to reuqest it be changed, it should change the value before returning true
- Returns:
- whether the value has been changed. true if changed, false if not
Reimplemented in NvSampleApp.
virtual void NvAppBase::initRendering | ( | void | ) | [inline, virtual] |
Initialize rendering.
Called once the GLES context and surface have been created and bound to the main thread. Called again if the context is lost and must be recreated.
bool NvAppBase::isExiting | ( | ) | [inline, protected] |
Exit query.
- Returns:
- true if the application is in the process of exiting, false if not.
bool NvAppBase::isMobilePlatform | ( | ) | [inline] |
Mobile platform query.
Convenience function for testing whether this is a "mobile" GPU platform
- Returns:
- true if the platform is mobile, false if it is desktop-class
virtual void NvAppBase::mainLoop | ( | ) | [virtual] |
Mainloop function.
The base class provides an implementation of the mainloop that calls the virtual "callbacks" in the block below marked MAINLOOP CALLBACKS. Leaving this function as implemented in the App base class allows the application to simply override the individual callbacks to implement their app behavior. However, apps can still copy the source of the App::mainLoop into their own override of the function and either modify it slightly or completely replace it.
Reimplemented in NvSampleApp.
uint64_t NvAppBase::queryPerformanceCounter | ( | ) |
Get high-performance timer value.
- Returns:
- the number of "ticks" since the system was started.
uint64_t NvAppBase::queryPerformanceCounterFrequency | ( | ) |
Get high-performance timer frequency.
- Returns:
- the number of "ticks" per second, used to convert queryPerformanceCounter to time.
virtual void NvAppBase::reshape | ( | int32_t | width, | |
int32_t | height | |||
) | [inline, virtual] |
Resize callback.
Called when the main rendering window changes dimensions and before the first frame is rendered after initRendering
- Parameters:
-
[in] width the new window width in pixels [in] height the new window height in pixels
void NvAppBase::setGLContext | ( | NvGLAppContext * | context | ) | [inline] |
Set GL context info.
- Parameters:
-
[in] context the GL context info
bool NvAppBase::showDialog | ( | const char * | title, | |
const char * | contents, | |||
bool | exitApp | |||
) |
Show info/error dialog.
Shows a dialog and, if desired, exits the app on dialog close
- Parameters:
-
[in] title a null-terminated string with the title for the dialog [in] contents a null-terminate string with the text to be shown in the dialog [in] exitApp if true, the app will exit when the dialog is closed. Useful for error dialogs
- Returns:
- true on success, false on failure
virtual void NvAppBase::shutdownRendering | ( | void | ) | [inline, virtual] |
Shutdown rendering.
Called when the GLES context has just been shut down; it indicates that all GL resources in the app's context have been deleted and are invalid, and will need to be recreated on the next call to initRendering. This function should also be used to shut down any secondary threads that generate GL calls such as buffer mappings.
Because the sequence of shutdownRendering/initRendering may be called without the app being completely shut down (e.g. lost context), the app needs to use this to delete non-GL resources (e.g. system memory related to 3D resources) and indicate that it needs to reload any GL resources on initRendering
virtual void NvAppBase::update | ( | void | ) | [inline, virtual] |
Application animation update.
Called at regular (frame-scale) intervals even if the app is not focused. Always called directly before a render, but will be called without a render if the app is not focused. Optional.
bool NvAppBase::writeLogFile | ( | const std::string & | path, | |
bool | append, | |||
const char * | fmt, | |||
... | ||||
) |
Write text to logging file.
Writes the given printf-like arguments to the specified log file.
- Parameters:
-
[in] path the partial path and filename to write. [in] append if true, the string is written to the end of the existing file. If false, the file is cleared and the string written to the top of the file [in] fmt printf-style varargs format string and following arguments
- Returns:
- true on success, false on failure.
bool NvAppBase::writeScreenShot | ( | int32_t | width, | |
int32_t | height, | |||
const uint8_t * | data, | |||
const std::string & | path | |||
) |
Write RGB image to file Debugging function to write a block of RGB 24-bit data to an image file.
Format and extension of the image file, as well as location is platform-specific.
- Parameters:
-
[in] width the width of the image to be written in pixels [in] height the height of the image to be written in pixels [in] data pointer to width*height*4 bytes with the RGBA image data. [in] path the partial (relative) path and filename (no extension) to be written with the pixel data.
- Returns:
- true on success and false on failure.
The documentation for this class was generated from the following file: