NvUISlider Class Reference
An interactive ValueBar with draggable thumb, for volume, play position, etc. More...
#include <NvUI.h>
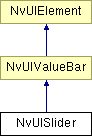
Public Member Functions | |
NvUISlider (NvUIGraphicFrame *emptybar, NvUIGraphicFrame *fullbar, NvUIGraphic *thumb, uint32_t actionCode) | |
Default constructor specializing on NvUIValueBar to make an interactive slider. | |
virtual | ~NvUISlider () |
Default destructor. | |
virtual NvUIEventResponse | HandleEvent (const NvGestureEvent &ev, NvUST timeUST, NvUIElement *hasFocus) |
Override for specialized input handling of dragging the thumb on the bar and generating reactions. | |
virtual NvUIEventResponse | HandleReaction (const NvUIReaction &react) |
Override for handling someone other than use send a reaction with our code as a way to pass along value changes. | |
virtual void | SetDimensions (float w, float h) |
Override to handle proxying to thumb element. | |
virtual void | SetOrigin (float x, float y) |
Override to handle proxying to thumb element. | |
virtual void | SetAlpha (float a) |
Override to handle proxying to thumb element. | |
virtual void | SetValue (float value) |
Override to have better control over when value really changes, when visual elements update. | |
virtual void | SetHitMargin (float hitwide, float hittall) |
Add extra horizontal/vertical margin to thumb hit rect to make it easier to grab. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Override to handle drawing thumb element over base valuebar, and optional value text output. | |
void | SetOutput (NvUIText *out, bool owns=false) |
Takes an NvUIText element to be updated live as our value changes. | |
void | SetSmoothScrolling (bool smooth) |
Set true to enable posting of reaction during movement of the thumb. | |
void | SetStepValue (float step) |
Set incremental 'steps' for value changes, like 'tick marks' on a ruler. | |
uint32_t | GetActionCode () |
Returns the action code we use when raising reaction to user interaction. | |
Protected Attributes | |
NvUIGraphic * | m_thumb |
The graphic drawn over the NvUIValueBar as the draggable 'thumb' piece. | |
bool | m_wasHit |
Used by HandleEvent and internal hit-tracking. | |
float | m_startValue |
The thumb 'value' cached at the start of a drag, in order to reset back on a 'failed' drag interaction. | |
float | m_stepValue |
The amount to step the value at a time while dragging the thumb -- 'tick marks'. | |
uint32_t | m_action |
The NvUIReaction code 'posted' by the event handler when the thumb is released in a valid position. | |
bool | m_smooth |
If set true, this causes constant reactions during live dragging of the thumb. | |
NvUIText * | m_output |
A textbox where we can print current value. | |
bool | m_ownsOutput |
Whether we own the textbox as 'part of us', or whether it's in some other container. |
Detailed Description
An interactive ValueBar with draggable thumb, for volume, play position, etc.This is a natural extension of the NvUIValueBar class, adding a graphical 'thumb' to the bar for both visual feedback and user interactive control over the value (such as page, zoom level, audio volume, playback position, or other similar input needs).
The NvUISlider is set up much like the NvUIValueBar, with the addition of passing in a NvUIGraphic for the thumb, and a unique NvUIReaction code to 'post' when the thumb has been dragged and released in a valid position. That Reaction can then be handled by any other UI element within the same 'tree' as the slider, perfect for notifying an upper-level 'container' object that something under it has changed and needs to be handled. This allows for a NvUISlider to be instantiated directly without subclassing if so desired.
- See also:
- NvUIElement
Constructor & Destructor Documentation
NvUISlider::NvUISlider | ( | NvUIGraphicFrame * | emptybar, | |
NvUIGraphicFrame * | fullbar, | |||
NvUIGraphic * | thumb, | |||
uint32_t | actionCode | |||
) |
Default constructor specializing on NvUIValueBar to make an interactive slider.
Adds an interactive 'thumb' on top of a value bar to allow the user to manually adjust its value.
- Parameters:
-
emptybar The NvUIGraphicFrame visual for the empty/unfilled bar background fullbar The NvUIGraphicFrame visual for the filled bar overlay thumb An NvUIGraphic for the draggable 'thumb' button that sits over the bar. actionCode The action code sent in a reaction when there has been a value change.
virtual NvUISlider::~NvUISlider | ( | ) | [virtual] |
Default destructor.
Member Function Documentation
virtual void NvUISlider::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
Override to handle drawing thumb element over base valuebar, and optional value text output.
Reimplemented from NvUIValueBar.
uint32_t NvUISlider::GetActionCode | ( | ) | [inline] |
Returns the action code we use when raising reaction to user interaction.
virtual NvUIEventResponse NvUISlider::HandleEvent | ( | const NvGestureEvent & | ev, | |
NvUST | timeUST, | |||
NvUIElement * | hasFocus | |||
) | [virtual] |
Override for specialized input handling of dragging the thumb on the bar and generating reactions.
Reimplemented from NvUIElement.
virtual NvUIEventResponse NvUISlider::HandleReaction | ( | const NvUIReaction & | react | ) | [virtual] |
Override for handling someone other than use send a reaction with our code as a way to pass along value changes.
Reimplemented from NvUIElement.
virtual void NvUISlider::SetAlpha | ( | float | a | ) | [virtual] |
virtual void NvUISlider::SetDimensions | ( | float | w, | |
float | h | |||
) | [virtual] |
virtual void NvUISlider::SetHitMargin | ( | float | hitwide, | |
float | hittall | |||
) | [virtual] |
Add extra horizontal/vertical margin to thumb hit rect to make it easier to grab.
virtual void NvUISlider::SetOrigin | ( | float | x, | |
float | y | |||
) | [virtual] |
void NvUISlider::SetOutput | ( | NvUIText * | out, | |
bool | owns = false | |||
) |
Takes an NvUIText element to be updated live as our value changes.
void NvUISlider::SetSmoothScrolling | ( | bool | smooth | ) | [inline] |
Set true to enable posting of reaction during movement of the thumb.
When disabled/false, we only post reaction when the thumb is released in its final position. When true, we update constantly while the thumb is being dragged. The default is false/disabled.
void NvUISlider::SetStepValue | ( | float | step | ) | [inline] |
Set incremental 'steps' for value changes, like 'tick marks' on a ruler.
This enforces a given stepping of values along the min/max range. Most helpful when the range is large, but you want specific 'stop' points.
virtual void NvUISlider::SetValue | ( | float | value | ) | [virtual] |
Override to have better control over when value really changes, when visual elements update.
Reimplemented from NvUIValueBar.
Member Data Documentation
uint32_t NvUISlider::m_action [protected] |
The NvUIReaction code 'posted' by the event handler when the thumb is released in a valid position.
NvUIText* NvUISlider::m_output [protected] |
A textbox where we can print current value.
bool NvUISlider::m_ownsOutput [protected] |
Whether we own the textbox as 'part of us', or whether it's in some other container.
bool NvUISlider::m_smooth [protected] |
If set true, this causes constant reactions during live dragging of the thumb.
Defaults false.
float NvUISlider::m_startValue [protected] |
The thumb 'value' cached at the start of a drag, in order to reset back on a 'failed' drag interaction.
float NvUISlider::m_stepValue [protected] |
The amount to step the value at a time while dragging the thumb -- 'tick marks'.
NvUIGraphic* NvUISlider::m_thumb [protected] |
The graphic drawn over the NvUIValueBar as the draggable 'thumb' piece.
bool NvUISlider::m_wasHit [protected] |
Used by HandleEvent
and internal hit-tracking.
Set when we had a successful press/click in the thumb, so we are then tracking for drag/flick/release.
The documentation for this class was generated from the following file: